用C语言写一个和chmod功能相同的程序,但不可以使用chmod函数
时间: 2024-10-21 08:07:00 浏览: 31
在C语言中,`chmod`函数用于改变文件或目录的权限,它直接操作了系统底层的功能。如果我们想要编写一个不使用`chmod`函数的程序,我们可以手动模拟计算新的权限值,并通过文件访问权限位来设置。这里我们以Unix/Linux的权限模式为例,文件权限分为用户、组和其他三个部分,每个部分又分读(r)、写(w)、执行(x)三种权限。
下面是一个简单的例子,该程序将接受一个新的权限模式作为输入,然后使用`open()`, `fchmod()`和`fclose()`等函数来实现类似`chmod`的效果:
```c
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
#define S_IRUSR (1 << 0) // 用户可读
#define S_IWUSR (1 << 1) // 用户可写
#define S_IXUSR (1 << 2) // 用户可执行
// ...其他权限定义...
int main(int argc, char *argv[]) {
if (argc != 3) {
printf("Usage: chmod_program file_path permission\n");
return 1;
}
char *file_path = argv[1];
int mode = strtol(argv[2], NULL, 8); // 将八进制权限字符串转为整数
int fd = open(file_path, O_RDONLY); // 打开文件以获取当前权限
if (fd == -1) {
perror("Opening file failed");
return 1;
}
// 获取当前权限
struct stat sb;
if (fstat(fd, &sb)) {
perror("Getting file status failed");
close(fd);
return 1;
}
close(fd);
// 设置新权限
mode_t new_mode = sb.st_mode; // 保留现有权限
new_mode &= ~S_IRWXU | ~S_IRWXG | ~S_IRWXO; // 清除原有权限
new_mode |= ((mode & 0o700) >> 6) << 6; // 设置用户权限
new_mode |= ((mode & 0o070) >> 3) << 3; // 设置组权限
new_mode |= (mode & 0o007); // 设置其他人权限
int result = chmod(file_path, new_mode);
if (result == -1) {
perror("Changing permissions failed");
return 1;
}
printf("Permission changed to %o for file '%s'\n", new_mode, file_path);
return 0;
}
```
在这个示例中,你需要自行处理权限模式的合法性检查以及错误处理。
阅读全文
相关推荐
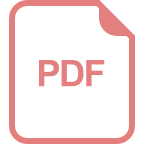
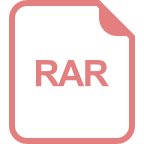
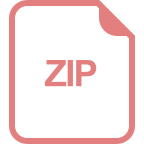











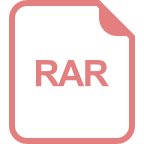
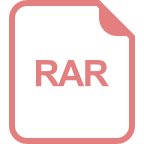
