用c++写出这个文档里要求的程序
时间: 2024-12-08 08:23:48 浏览: 5
为了满足《数据结构-实验三》的要求,下面提供了C++代码示例,包括顺序栈、链栈、循环队列和链队列的基本操作。
### 1. 顺序栈
```cpp
#include <iostream>
#define MAX_SIZE 100
class SeqStack {
private:
int data[MAX_SIZE];
int top;
public:
SeqStack() : top(-1) {}
bool isFull() const {
return top == MAX_SIZE - 1;
}
bool isEmpty() const {
return top == -1;
}
void push(int x) {
if (isFull()) {
std::cout << "Stack overflow" << std::endl;
return;
}
data[++top] = x;
}
int pop() {
if (isEmpty()) {
std::cout << "Stack underflow" << std::endl;
return -1;
}
return data[top--];
}
int getTop() const {
if (isEmpty()) {
std::cout << "Stack is empty" << std::endl;
return -1;
}
return data[top];
}
};
int main() {
SeqStack stack;
int elements[] = {1, 5, 2, 3, 4};
for (int elem : elements) {
stack.push(elem);
}
while (!stack.isEmpty()) {
std::cout << stack.pop() << " ";
}
std::cout << std::endl;
return 0;
}
```
### 2. 链栈
```cpp
#include <iostream>
struct Node {
int data;
Node* next;
};
class LinkStack {
private:
Node* top;
public:
LinkStack() : top(nullptr) {}
~LinkStack() {
while (top != nullptr) {
Node* temp = top;
top = top->next;
delete temp;
}
}
bool isEmpty() const {
return top == nullptr;
}
void push(int x) {
Node* newNode = new Node{ x, top };
top = newNode;
}
int pop() {
if (isEmpty()) {
std::cout << "Stack underflow" << std::endl;
return -1;
}
Node* temp = top;
int value = temp->data;
top = top->next;
delete temp;
return value;
}
int getTop() const {
if (isEmpty()) {
std::cout << "Stack is empty" << std::endl;
return -1;
}
return top->data;
}
};
int main() {
LinkStack stack;
int elements[] = {1, 5, 2, 3, 4};
for (int elem : elements) {
stack.push(elem);
}
while (!stack.isEmpty()) {
std::cout << stack.pop() << " ";
}
std::cout << std::endl;
return 0;
}
```
### 3. 循环队列
```cpp
#include <iostream>
#define MAX_SIZE 6
class CircularQueue {
private:
int data[MAX_SIZE];
int front, rear;
public:
CircularQueue() : front(0), rear(0) {}
bool isEmpty() const {
return front == rear;
}
bool isFull() const {
return (rear + 1) % MAX_SIZE == front;
}
void enqueue(int x) {
if (isFull()) {
std::cout << "Queue overflow" << std::endl;
return;
}
data[rear] = x;
rear = (rear + 1) % MAX_SIZE;
}
int dequeue() {
if (isEmpty()) {
std::cout << "Queue underflow" << std::endl;
return -1;
}
int value = data[front];
front = (front + 1) % MAX_SIZE;
return value;
}
int getFront() const {
if (isEmpty()) {
std::cout << "Queue is empty" << std::endl;
return -1;
}
return data[front];
}
};
int main() {
CircularQueue queue;
int elements[] = {1, 2, 3, 4, 5};
for (int elem : elements) {
queue.enqueue(elem);
}
while (!queue.isEmpty()) {
std::cout << queue.dequeue() << " ";
}
std::cout << std::endl;
return 0;
}
```
### 4. 链队列
```cpp
#include <iostream>
struct Node {
int data;
Node* next;
};
class LinkQueue {
private:
Node* front;
Node* rear;
public:
LinkQueue() : front(nullptr), rear(nullptr) {}
~LinkQueue() {
while (front != nullptr) {
Node* temp = front;
front = front->next;
delete temp;
}
}
bool isEmpty() const {
return front == nullptr;
}
void enqueue(int x) {
Node* newNode = new Node{ x, nullptr };
if (isEmpty()) {
front = rear = newNode;
} else {
rear->next = newNode;
rear = newNode;
}
}
int dequeue() {
if (isEmpty()) {
std::cout << "Queue underflow" << std::endl;
return -1;
}
Node* temp = front;
int value = temp->data;
front = front->next;
if (front == nullptr) {
rear = nullptr;
}
delete temp;
return value;
}
int getFront() const {
if (isEmpty()) {
std::cout << "Queue is empty" << std::endl;
return -1;
}
return front->data;
}
};
int main() {
LinkQueue queue;
int elements[] = {1, 2, 3, 4, 5};
for (int elem : elements) {
queue.enqueue(elem);
}
while (!queue.isEmpty()) {
std::cout << queue.dequeue() << " ";
}
std::cout << std::endl;
return 0;
}
```
这些代码实现了文档中要求的所有功能,并且可以通过提供的验收/测试用例进行验证。希望这对你有帮助!
阅读全文
相关推荐
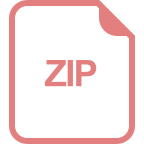
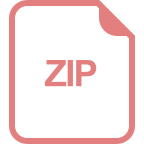
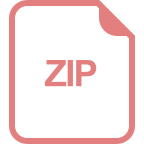
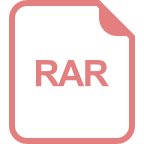
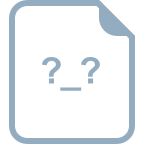
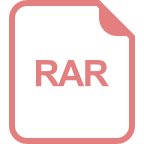
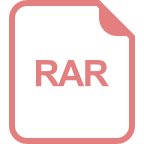
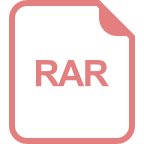
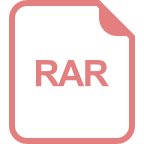
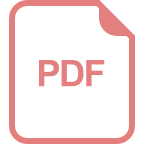
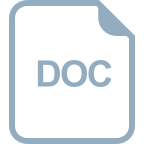
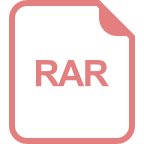
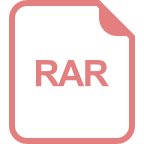
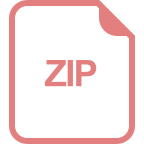
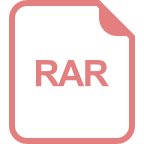
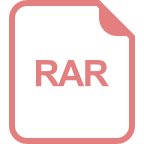
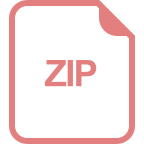
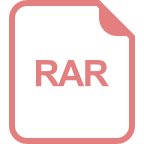
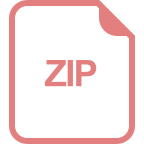