编写一个 Java 应用程序,从键盘输入一个x值,程序输出y的值。
时间: 2024-09-24 13:12:16 浏览: 12
在Java中编写一个简单的应用程序,可以利用多态的概念来处理这个需求。假设我们有一个`Shape`接口和两个实现了这个接口的具体类`Circle`和`Rectangle`,它们分别有自己的计算面积的方法。用户输入的x值可能是半径、宽度和高度之一。以下是示例代码:
```java
import java.util.Scanner;
// 定义Shape接口
interface Shape {
double getArea();
}
// 实现Shape接口的类
class Circle implements Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
@Override
public double getArea() {
return Math.PI * radius * radius;
}
}
class Rectangle implements Shape {
private double width, height;
public Rectangle(double width, double height) {
this.width = width;
this.height = height;
}
@Override
public double getArea() {
return width * height;
}
}
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("请输入几何图形(circle或rectangle),然后按Enter键:");
String shapeType = scanner.nextLine();
Shape shape;
if ("circle".equals(shapeType)) {
System.out.println("请输入圆的半径:");
double radius = scanner.nextDouble();
shape = new Circle(radius);
} else if ("rectangle".equals(shapeType)) {
System.out.println("请输入矩形的宽度和高度:");
double width = scanner.nextDouble(), height = scanner.nextDouble();
shape = new Rectangle(width, height);
} else {
System.out.println("无效的形状类型");
return;
}
// 输出形状的面积
double area = shape.getArea();
System.out.println("该图形的面积是: " + area);
}
}
相关推荐
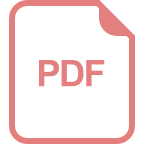
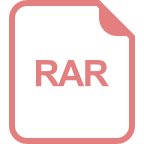
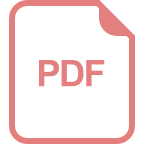














