假设我现在有一个前端界面,表单中有一个邮件框,后面有一个发送验证码的按钮,触发该按钮发送当前页面的邮箱号到@controler标记的类中被@RequestMapping标记的方法接受该邮箱号通过AJAX怎么写
时间: 2024-09-25 14:19:33 浏览: 44
首先,你需要在HTML页面上设置好表单,包括邮箱输入框和发送验证码按钮。在JavaScript部分,你可以使用AJAX技术来异步向服务器请求发送验证码。下面是一个基本的例子:
```html
<!-- HTML部分 -->
<form id="sendCodeForm">
<input type="email" id="emailInput" placeholder="请输入邮箱">
<button type="button" onclick="sendVerificationCode()">发送验证码</button>
</form>
<script>
function sendVerificationCode() {
var email = document.getElementById('emailInput').value;
// 创建XMLHttpRequest对象
var xhr = new XMLHttpRequest();
// 设置请求方法和URL
xhr.open('POST', '/api/sendVerificationCode', true);
// 设置请求头,如果需要的话,可以包含Content-Type
xhr.setRequestHeader("Content-Type", "application/x-www-form-urlencoded");
// 当请求完成时执行回调函数
xhr.onload = function() {
if (xhr.status === 200) {
console.log('验证码已发送');
} else {
console.error('发送验证码失败');
}
};
// 发送数据
xhr.send('email=' + encodeURIComponent(email));
}
</script>
```
在这个例子中,当用户点击“发送验证码”按钮时,`sendVerificationCode`函数会被触发,它会从邮箱输入框获取值,并将其编码成字符串发送到`/api/sendVerificationCode`这个`@RequestMapping`标注的方法。
在Spring MVC框架中,对应的Controller类可能会像这样:
```java
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class VerificationCodeController {
@PostMapping("/api/sendVerificationCode")
public String sendVerificationCode(@RequestParam("email") String email) {
// 实现发送验证码的逻辑,这里只是一个示例
// 需要将email保存并发送验证码给指定邮箱
System.out.println("接收到的邮箱地址:" + email);
// 实际操作如:发送邮件、数据库记录等
return "success";
}
}
```
请注意,这只是一个简化的示例,实际应用中还需要处理错误、验证电子邮件格式以及添加适当的异常处理。另外,如果你使用了前端框架(如Vue.js或React),则发送请求的方式可能会有所不同。
相关推荐
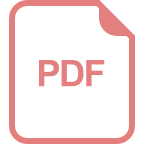
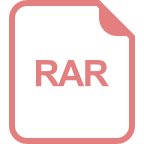
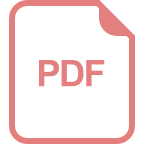
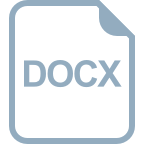
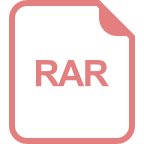
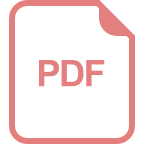
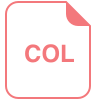
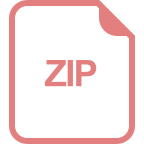
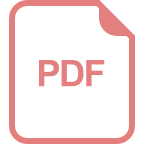
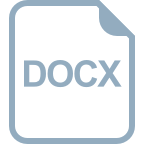
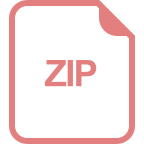
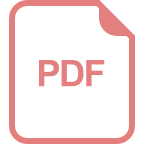
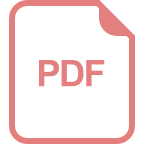
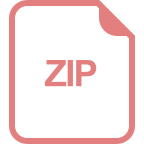
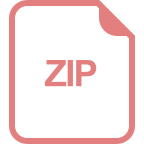