帮我用c语言完成一下任务:建立一个包含 10 个结点的动态链表,每个结点包括书号、书名、作者、出版社和价格。输 入一个书名,如果链表中的结点所包含的书名等于输入的书名,则将此结点删去。
时间: 2024-12-21 22:26:07 浏览: 3
在C语言中,我们可以使用结构体定义链表节点,并使用头指针来管理链表。这里是一个简单的示例程序,实现了创建链表、插入节点、查找并删除节点的功能:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义链表节点结构
typedef struct BookNode {
int bookNo;
char title[50];
char author[50];
char publisher[50];
float price;
struct BookNode* next;
} BookNode;
// 创建新节点
BookNode* createNode(int no, char* title, char* author, char* publisher, float price) {
BookNode* newNode = (BookNode*)malloc(sizeof(BookNode));
if (!newNode) {
printf("Memory allocation failed.\n");
return NULL;
}
newNode->bookNo = no;
strcpy(newNode->title, title);
strcpy(newNode->author, author);
strcpy(newNode->publisher, publisher);
newNode->price = price;
newNode->next = NULL;
return newNode;
}
// 插入节点到链表
void insertNode(BookNode** head, BookNode* newNode) {
if (*head == NULL) {
*head = newNode;
} else {
BookNode* temp = *head;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = newNode;
}
}
// 查找并删除匹配的节点
void deleteNode(BookNode** head, const char* targetTitle) {
if (*head == NULL) {
return;
}
BookNode* current = *head, *prev = NULL;
while (current != NULL && strcmp(current->title, targetTitle) != 0) {
prev = current;
current = current->next;
}
if (current != NULL) { // 找到了目标节点
if (prev == NULL) { // 如果是最开始的节点
*head = current->next;
} else {
prev->next = current->next;
}
free(current); // 释放内存
}
}
int main() {
BookNode* head = NULL;
// 创建10个节点并插入链表...
for (int i = 1; i <= 10; i++) {
char title[50];
printf("Enter book %d details (title): ", i);
scanf("%s", title);
char author[50], publisher[50];
float price;
printf("Author: ");
scanf("%s", author);
printf("Publisher: ");
scanf("%s", publisher);
printf("Price: ");
scanf("%f", &price);
BookNode* newNode = createNode(i, title, author, publisher, price);
insertNode(&head, newNode);
}
// 输入书名进行查找和删除操作
char searchTitle[50];
printf("Enter a title to find and remove: ");
scanf("%s", searchTitle);
deleteNode(&head, searchTitle);
// 输出链表中的所有书籍(已删除目标书)
BookNode* temp = head;
while (temp != NULL) {
printf("Book No: %d, Title: %s, Author: %s, Publisher: %s, Price: %.2f\n",
temp->bookNo, temp->title, temp->author, temp->publisher, temp->price);
temp = temp->next;
}
return 0;
}
```
这个程序首先创建一个链表,然后让用户输入书籍信息并添加到链表中。接着用户可以输入一个书名,程序会找到并删除链表中相应的节点。最后,链表的内容会被打印出来。
阅读全文
相关推荐
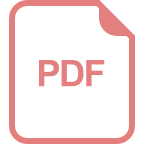
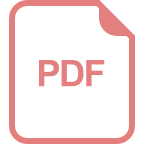



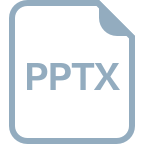
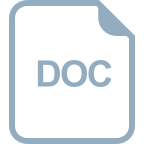
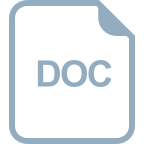
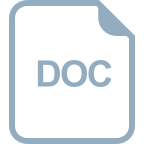
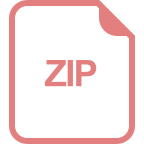
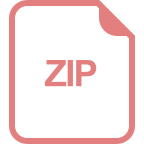
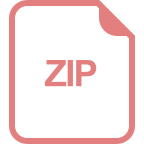
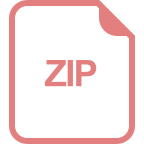
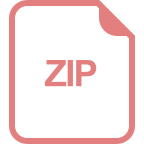