请帮我用python写标签平滑的caffer层
时间: 2023-09-27 17:07:03 浏览: 39
标签平滑(label smoothing)是一种用于减少过拟合的技术,它通过将真实标签与一些平滑的伪标签混合来训练模型。在Caffe中,可以通过自定义层来实现标签平滑。下面是一个使用Python实现标签平滑的Caffe层的示例代码:
```python
import caffe
import numpy as np
class LabelSmoothingLayer(caffe.Layer):
def setup(self, bottom, top):
if len(bottom) != 2:
raise Exception("Need two inputs to compute label smoothing loss.")
# 检查输入数据维度是否匹配
if bottom[0].count != bottom[1].count:
raise Exception("Inputs must have the same dimension.")
self.smooth = 0.1 # 平滑因子
self.softmax = np.zeros_like(bottom[0].data, dtype=np.float32)
def reshape(self, bottom, top):
top[0].reshape(1)
def forward(self, bottom, top):
# 计算平滑的伪标签
self.softmax[...] = bottom[0].data
self.softmax -= np.max(self.softmax, axis=1, keepdims=True)
self.softmax = np.exp(self.softmax)
self.softmax /= np.sum(self.softmax, axis=1, keepdims=True)
self.smooth_labels = (1 - self.smooth) * self.softmax + self.smooth / bottom[0].shape[1]
# 计算交叉熵损失
self.loss = -np.sum(self.smooth_labels * np.log(bottom[1].data + 1e-10)) / bottom[0].shape[0]
top[0].data[...] = self.loss
def backward(self, top, propagate_down, bottom):
if propagate_down:
bottom[0].diff[...] = (self.smooth_labels - bottom[1].data) / bottom[0].shape[0]
bottom[1].diff[...] = -self.smooth_labels / (bottom[0].shape[0] * (bottom[1].data + 1e-10))
```
在上面的代码中,我们定义了一个名为LabelSmoothingLayer的自定义层,实现了标签平滑。在setup()函数中,我们首先检查输入的数据维度是否匹配,然后初始化了smooth和softmax变量。在reshape()函数中,我们指定输出数据的维度。在forward()函数中,我们计算出平滑的伪标签并计算交叉熵损失,并将损失值保存到top[0]中。在backward()函数中,我们计算梯度,并将梯度值保存到bottom[0]和bottom[1]中。
需要注意的是,在计算梯度时,我们在分母中加上了一个小的常数1e-10,这是为了避免分母为0的情况。
相关推荐
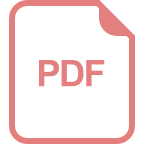














