c语言删除字符串数组中的字符串
时间: 2023-10-20 08:05:56 浏览: 217
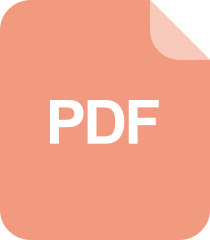
C语言之从字符数组中删除特定的字符
删除字符串数组中的字符串可以通过以下步骤实现:
1. 定义一个新的字符串数组,用于存放删除指定字符串后的结果。
2. 遍历原字符串数组,对于不需要删除的字符串,将其复制到新的字符串数组中。
3. 对于需要删除的字符串,可以直接跳过不复制到新的字符串数组中。
4. 最后将新的字符串数组复制回原来的字符串数组中,完成删除操作。
以下是一个示例代码:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str[5][20] = {"hello", "world", "c", "language", "program"};
int len = 5;
char del_str[] = "c";
// 创建新的字符串数组
char new_str[5][20];
int new_len = 0;
// 遍历原字符串数组,复制不需要删除的字符串
for (int i = 0; i < len; i++) {
if (strcmp(str[i], del_str) == 0) {
// 如果需要删除,则跳过
continue;
}
strcpy(new_str[new_len++], str[i]);
}
// 将新的字符串数组复制回原来的字符串数组中
for (int i = 0; i < new_len; i++) {
strcpy(str[i], new_str[i]);
}
len = new_len;
// 输出删除后的结果
for (int i = 0; i < len; i++) {
printf("%s ", str[i]);
}
printf("\n");
return 0;
}
```
输出结果为:
```
hello world language program
```
阅读全文
相关推荐


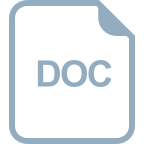
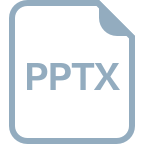
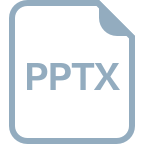
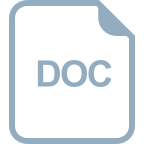







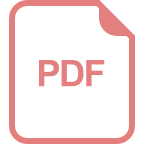

