libolecf怎么用
时间: 2023-12-01 16:58:48 浏览: 50
libolecf是一个用于读取OLE2复合文件格式(如Microsoft Office文档)的库。以下是使用libolecf的基本步骤:
1. 引入头文件
```c++
#include <libolecf.h>
```
2. 打开OLE2复合文件
```c++
IOlecfFile* file = nullptr;
auto error = IOlecfFile_open(L"example.docx", &file);
if (error != IOLECF_OK) {
// 打开文件失败
}
```
3. 获取根目录
```c++
IOlecfDirectory* root = nullptr;
error = IOlecfFile_get_root_directory(file, &root);
if (error != IOLECF_OK) {
// 获取根目录失败
}
```
4. 遍历目录树
可以使用`IOlecfDirectory_enumerate_tree`函数遍历目录树,例如:
```c++
void enumerate_directory(IOlecfDirectory* directory, int depth) {
IOlecfEnumerator* enumerator = nullptr;
auto error = IOlecfDirectory_enumerate_tree(directory, &enumerator);
if (error != IOLECF_OK) {
// 枚举目录失败
return;
}
IOlecfItem* item = nullptr;
while (IOlecfEnumerator_get_next(enumerator, &item) == IOLECF_OK) {
// 输出当前项的名称和深度
LPOLESTR name = nullptr;
IOlecfItem_get_name(item, &name);
wprintf(L"%*s%s\n", depth * 4, L"", name);
// 如果当前项是目录,则递归枚举其子目录
if (IOlecfItem_is_directory(item)) {
IOlecfDirectory* subdirectory = nullptr;
IOlecfItem_get_directory(item, &subdirectory);
enumerate_directory(subdirectory, depth + 1);
IOlecfDirectory_release(subdirectory);
}
IOlecfItem_release(item);
}
IOlecfEnumerator_release(enumerator);
}
enumerate_directory(root, 0);
```
以上代码会输出目录树的结构。
5. 读取文件内容
可以使用`IOlecfItem_open_stream`函数读取文件内容,例如:
```c++
IOlecfItem* item = nullptr;
error = IOlecfDirectory_get_item_by_name(root, L"word/document.xml", &item);
if (error == IOLECF_OK) {
IStream* stream = nullptr;
error = IOlecfItem_open_stream(item, &stream);
if (error == IOLECF_OK) {
// 读取流内容
// ...
// 关闭流
IStream_Release(stream);
}
IOlecfItem_release(item);
}
```
以上代码会打开example.docx中的word/document.xml文件,并读取其内容。
注意:以上代码仅作为示例,实际使用时应该检查每个函数的返回值,并在出错时释放已分配的资源。
相关推荐
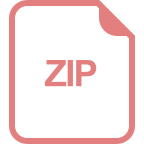









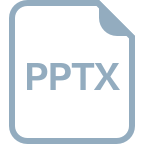