tensorflow检查gpu的代码
时间: 2023-10-04 21:09:02 浏览: 41
你可以使用以下代码来检查 TensorFlow 是否正确识别了 GPU:
```python
import tensorflow as tf
# 创建一个 TensorFlow 的会话
sess = tf.Session()
# 打印 TensorFlow 版本和 GPU 是否可用
print("TensorFlow 版本:", tf.__version__)
print("GPU 可用:", tf.test.is_gpu_available())
# 打印当前可用的 GPU 设备列表
gpu_devices = tf.config.experimental.list_physical_devices('GPU')
print("可用的 GPU 设备列表:", gpu_devices)
# 打印当前默认的 GPU 设备
print("默认的 GPU 设备:", tf.test.gpu_device_name())
# 关闭 TensorFlow 会话
sess.close()
```
运行这段代码后,你应该能够看到 TensorFlow 版本、GPU 的可用性以及当前可用的 GPU 设备列表和默认的 GPU 设备名称。如果 GPU 可用并且正确识别,你将看到相关的信息。如果没有找到 GPU 或者 TensorFlow 没有正确配置 GPU,你将看到相应的提示信息。
请注意,在运行此代码之前,确保已经正确安装了 TensorFlow 和相应的 GPU 驱动程序以及 CUDA 和 cuDNN。
相关问题
tensorflow启动gpu代码
要在TensorFlow中启用GPU支持,需要按照以下步骤进行操作:
1. 确保你已经正确安装了NVIDIA驱动和CUDA。你可以通过运行`nvidia-smi`命令来检查NVIDIA驱动是否正确安装,并通过运行`nvcc --version`命令来检查CUDA是否正确安装。
2. 安装TensorFlow GPU版本。你可以使用以下命令安装最新版本的TensorFlow GPU:
```
pip install tensorflow-gpu
```
3. 在代码中添加以下内容以启用GPU支持:
```python
import tensorflow as tf
physical_devices = tf.config.list_physical_devices('GPU')
tf.config.experimental.set_memory_growth(physical_devices[0], True)
```
这将启用TensorFlow的GPU支持并允许动态分配GPU内存。
4. 在你的代码中使用TensorFlow时,TensorFlow将自动使用可用的GPU来加速计算。你可以通过以下方式验证GPU是否正常工作:
```python
import tensorflow as tf
print("Num GPUs Available: ", len(tf.config.list_physical_devices('GPU')))
```
这将打印出可用的GPU数量。
请注意,以上步骤假设你已经正确安装了NVIDIA驱动和CUDA,并且你的系统上有可用的GPU。如果你遇到任何问题,请检查你的环境设置和硬件配置。
tensorflow调用gpu代码
要使用TensorFlow调用GPU,你可以按照以下步骤进行操作:
1. 首先,你可以使用以下代码检查你的系统上有多少个GPU和CPU可用:
```
import tensorflow as tf
gpus = tf.config.experimental.list_physical_devices(device_type='GPU')
cpus = tf.config.experimental.list_physical_devices(device_type='CPU')
print(gpus, cpus)
```
这将返回可用的GPU和CPU的列表。
2. 如果你想启用GPU加速,你可以使用以下代码:
```
import tensorflow as tf
# 创建会话对象并启动TensorFlow运行时环境
sess = tf.Session()
# 设置TensorFlow的默认设备为GPU
with tf.device('/gpu:0'):
# 在此处定义你的计算图和操作
```
这将在GPU上执行你的计算图和操作。
3. 如果你想限制GPU的使用和内存大小,你可以使用以下代码:
```
import tensorflow as tf
gpus = tf.config.experimental.list_physical_devices(device_type='GPU')
tf.config.experimental.set_virtual_device_configuration(gpus<span class="em">1</span><span class="em">2</span><span class="em">3</span><span class="em">4</span>
相关推荐
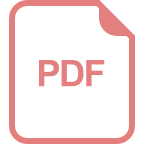
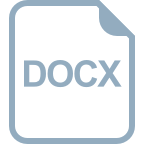












