java 开发国标gb28181代码
时间: 2023-08-10 18:02:16 浏览: 175
实现 GB28181 标准的视频监控系统,需要涉及到很多领域的知识,包括网络通信、视频编解码、设备管理等。下面给出一个简单的 Java 代码示例,用于实现基本的设备管理和视频流传输功能:
1. 引入相关依赖库
```java
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.boot.autoconfigure.jdbc.DataSourceAutoConfiguration;
import org.springframework.context.annotation.Bean;
import org.springframework.web.client.RestTemplate;
@SpringBootApplication(exclude = {DataSourceAutoConfiguration.class})
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
// 配置 RestTemplate
@Bean
public RestTemplate restTemplate() {
return new RestTemplate();
}
}
```
2. 实现设备管理功能
```java
import java.util.List;
public interface DeviceService {
// 获取设备列表
List<Device> getDeviceList();
// 获取设备信息
Device getDeviceInfo(String deviceId);
// 添加设备
boolean addDevice(Device device);
// 删除设备
boolean deleteDevice(String deviceId);
// 修改设备信息
boolean updateDevice(Device device);
}
```
```java
import org.springframework.stereotype.Service;
import java.util.ArrayList;
import java.util.List;
@Service
public class DeviceServiceImpl implements DeviceService {
// 模拟设备列表数据
private static List<Device> deviceList = new ArrayList<>();
static {
deviceList.add(new Device("device001", "192.168.1.100", 8000, "admin", "123456"));
deviceList.add(new Device("device002", "192.168.1.101", 8000, "admin", "123456"));
deviceList.add(new Device("device003", "192.168.1.102", 8000, "admin", "123456"));
}
@Override
public List<Device> getDeviceList() {
return deviceList;
}
@Override
public Device getDeviceInfo(String deviceId) {
for (Device device : deviceList) {
if (device.getDeviceId().equals(deviceId)) {
return device;
}
}
return null;
}
@Override
public boolean addDevice(Device device) {
if (getDeviceInfo(device.getDeviceId()) != null) {
return false;
}
deviceList.add(device);
return true;
}
@Override
public boolean deleteDevice(String deviceId) {
for (Device device : deviceList) {
if (device.getDeviceId().equals(deviceId)) {
deviceList.remove(device);
return true;
}
}
return false;
}
@Override
public boolean updateDevice(Device device) {
Device oldDevice = getDeviceInfo(device.getDeviceId());
if (oldDevice == null) {
return false;
}
oldDevice.setIp(device.getIp());
oldDevice.setPort(device.getPort());
oldDevice.setUsername(device.getUsername());
oldDevice.setPassword(device.getPassword());
return true;
}
}
```
3. 实现视频流传输功能
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.client.RestTemplate;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
@Controller
public class VideoController {
@Autowired
private RestTemplate restTemplate;
// 获取视频流
@GetMapping("/video/{deviceId}")
public void getVideoStream(@PathVariable("deviceId") String deviceId, HttpServletResponse response) {
Device device = deviceService.getDeviceInfo(deviceId);
if (device == null) {
response.setStatus(HttpServletResponse.SC_NOT_FOUND);
return;
}
String url = "http://" + device.getIp() + ":" + device.getPort() + "/video";
try {
byte[] videoData = restTemplate.getForObject(url, byte[].class);
response.getOutputStream().write(videoData);
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
这里的实现仅为示例,实际开发中需要根据具体需求进行更详细的代码编写和测试。
阅读全文
相关推荐
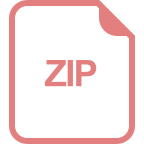
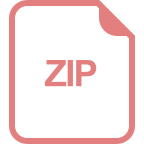
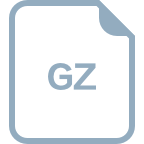

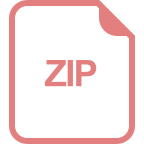
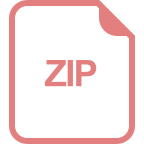
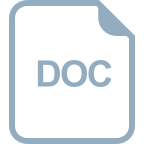
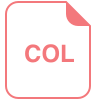
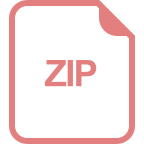
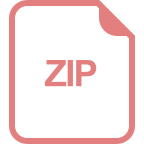
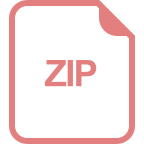
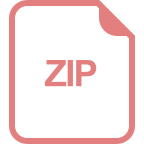
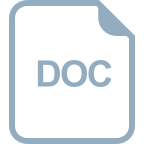
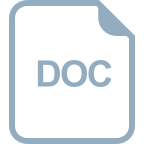
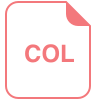
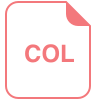
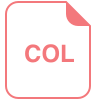
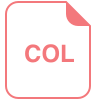