用单片机设计一个8路抢答器
时间: 2024-05-03 14:19:28 浏览: 9
设计一个8路抢答器的单片机程序需要以下步骤:
1. 确定硬件连接方式:使用一个IO口控制8个LED灯,使用另外8个IO口连接8个按钮。
2. 初始化程序:设置IO口的方向(输入或输出),初始化各个变量。
3. 进入主循环:在主循环中,不断扫描8个按钮的状态,如果有任何一个按钮被按下,就记录该按钮的编号,然后关闭其他按钮的输入。如果没有按钮被按下,则等待下一次扫描。
4. 抢答结束:抢答结束后,关闭所有按钮的输入,并打开所有LED灯。
下面是一个基本的程序框架示例,其中使用P1口作为LED输出口,P2口作为按钮输入口:
```
#include <reg52.h>
sbit LED1 = P1^0;
sbit LED2 = P1^1;
sbit LED3 = P1^2;
sbit LED4 = P1^3;
sbit LED5 = P1^4;
sbit LED6 = P1^5;
sbit LED7 = P1^6;
sbit LED8 = P1^7;
sbit BTN1 = P2^0;
sbit BTN2 = P2^1;
sbit BTN3 = P2^2;
sbit BTN4 = P2^3;
sbit BTN5 = P2^4;
sbit BTN6 = P2^5;
sbit BTN7 = P2^6;
sbit BTN8 = P2^7;
void main()
{
int i;
int winner = 0;
int btn_pressed = 0;
P1 = 0xff; // set all LEDs off
P2 = 0xff; // set all buttons input
while (1) {
// scan all buttons
if (!BTN1) {
btn_pressed = 1;
winner = 1;
} else if (!BTN2) {
btn_pressed = 1;
winner = 2;
} else if (!BTN3) {
btn_pressed = 1;
winner = 3;
} else if (!BTN4) {
btn_pressed = 1;
winner = 4;
} else if (!BTN5) {
btn_pressed = 1;
winner = 5;
} else if (!BTN6) {
btn_pressed = 1;
winner = 6;
} else if (!BTN7) {
btn_pressed = 1;
winner = 7;
} else if (!BTN8) {
btn_pressed = 1;
winner = 8;
}
// if any button is pressed, turn off other buttons and light winner LED
if (btn_pressed) {
for (i = 1; i <= 8; i++) {
if (i != winner) {
P2 |= (1 << (i-1));
}
}
P1 &= ~(1 << (winner-1));
break;
}
}
// all buttons off and all LEDs on
P1 = 0xff;
P2 = 0xff;
while (1) {}; // wait forever
}
```
这个程序使用了一个while循环来不断扫描按钮的状态,并通过P1口控制LED灯的状态。当任何一个按钮被按下时,记录该按钮的编号,关闭其他按钮的输入,然后打开该按钮对应的LED灯。抢答结束后,关闭所有按钮的输入,并打开所有LED灯。
相关推荐
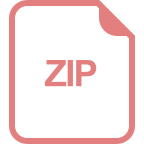














