<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Login Page</title> <style> .container { width: 400px; height: 300px; background-color: #fefefe; border: 1px solid #ccc; margin: 0 auto; padding: 20px; } .input-group { margin-bottom: 10px; } .input-group label { display: block; margin-bottom: 5px; } .input-group input, select { width: 100%; padding: 5px; border: 1px solid #ccc; border-radius: 4px; box-sizing: border-box; } .login-btn { background-color: #4CAF50; color: #fff; padding: 10px 20px; border: none; border-radius: 4px; cursor: pointer; float: right; } .login-btn:hover { background-color: #45a049; } </style> <script> function showAdminInput() { var userType = document.getElementById("user-type").value; if (userType == "admin") { document.getElementById("username-label").innerHTML = "请输入管理员用户名:"; } else { document.getElementById("username-label").innerHTML = "请输入用户名:"; } } function login() { var username = document.getElementById("username").value; var password = document.getElementById("password").value; var userType = document.getElementById("user-type").value; } </script> </head> <body> <div class="container" > <div class="input-group"> <label id="username-label">请输入用户名:</label> <input type="text" id="username" name="username"> </div> <div class="input-group"> <label>请输入密码:</label> <input type="password" id="password" name="password"> </div> <div class="input-group"> <label>请选择用户类型:</label> <select id="user-type" name="user-type" onchange="showAdminInput()"> <option value="normal">普通用户</option> <option value="admin">管理员</option> </select> </div> <button class="login-btn" onclick="login()">登录</button> </div> </body> </html>如何在这个鹿鸣添加一个图片作为整个网页的背景
时间: 2023-07-19 07:37:05 浏览: 44
您可以通过 CSS 样式来设置网页的背景图片。具体操作如下:
1. 在 CSS 文件或样式标签中,添加如下样式:
```
body {
background-image: url('图片路径');
background-size: cover; /* 可选,根据需要设置 */
background-position: center center; /* 可选,根据需要设置 */
background-repeat: no-repeat; /* 可选,根据需要设置 */
}
```
其中,`body` 表示网页的主体部分,`background-image` 属性值为图片的路径,可以是相对路径或绝对路径。例如,如果图片在项目根目录下的 img 文件夹内,路径可以设置为 `url('../img/图片名.jpg')`。
2. 将样式文件引入 HTML 文件或将样式标签添加到 HTML 文件的 head 标签中。
完成上述操作后,网页的背景就会被设置为指定的图片。注意,如果网页中有其他元素,如导航栏或内容区域等,它们可能会覆盖部分背景图片。如果需要调整背景图的显示方式,可以通过 CSS 的 `background-position` 和 `background-repeat` 属性来设置。
此外,您需要在 HTML 文件中添加图片元素来加载图片,例如:
```
<img src="图片路径" alt="背景图片">
```
但是,如果您想要设置整个网页的背景图片,建议仅使用 CSS 样式来设置背景图片,而不是添加图片元素。
相关问题
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <title>登录界面</title> </head> <body> <!-- 登录界面 --> <div id="page_login"> <div class="loginContainer"> <div style="font-size: 36px;margin-bottom: 20px;text-align: center;font-family: 'Lucida Sans', 'Lucida Sans Regular', 'Lucida Grande', 'Lucida Sans Unicode', Geneva, Verdana, sans-serif;"> Login</div> <input type="text" name="account" id="account" placeholder="account"> <input type="password" name="password" id="password" placeholder="password"> <button id="loginButton" onclick="getLogin()">login</button> </div> </div> </body> <script type="text/javascript" src="./js/index.js"></script> <script src="./js/jquery-3.3.1.js"></script> </html>
好的,以下是美化后的代码,使用了一些基本的CSS样式和布局:
```
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<title>登录界面</title>
<style>
body {
margin: 0;
padding: 0;
background-color: #f2f2f2;
font-family: 'Lucida Sans', 'Lucida Sans Regular', 'Lucida Grande', 'Lucida Sans Unicode', Geneva, Verdana, sans-serif;
}
#page_login {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
.loginContainer {
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
background-color: white;
border-radius: 5px;
box-shadow: 0px 0px 10px rgba(0,0,0,0.2);
padding: 30px;
}
.loginContainer > div {
font-size: 36px;
margin-bottom: 20px;
text-align: center;
}
input[type="text"], input[type="password"] {
width: 100%;
padding: 10px;
margin-bottom: 10px;
border: none;
border-radius: 5px;
box-shadow: 0px 0px 5px rgba(0,0,0,0.1);
}
#loginButton {
background-color: #007bff;
color: white;
padding: 10px 20px;
border: none;
border-radius: 5px;
box-shadow: 0px 0px 5px rgba(0,0,0,0.1);
transition: all 0.2s ease-in-out;
}
#loginButton:hover {
transform: scale(1.05);
box-shadow: 0px 0px 10px rgba(0,0,0,0.2);
}
</style>
</head>
<body>
<!-- 登录界面 -->
<div id="page_login">
<div class="loginContainer">
<div>Login</div>
<input type="text" name="account" id="account" placeholder="account">
<input type="password" name="password" id="password" placeholder="password">
<button id="loginButton" onclick="getLogin()">login</button>
</div>
</div>
</body>
<script type="text/javascript" src="./js/index.js"></script>
<script src="./js/jquery-3.3.1.js"></script>
</html>
```
根据这个页面制作一个js完善功能<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>购物车</title> <link rel="stylesheet" href="style.css"> <script src="script.js"></script> </head> <body> <header> <h1>欢迎来到我们的网站!</h1> <nav> <ul> <li><a href="index.html">首页</a></li> <li><a href="details_page.html">商品</a></li> <li><a href="cart.html">购物车</a></li> <li><a href="login.html">登录</a></li> </ul> </nav> </header> <main> <h2>购物车</h2> <ul> <li> <img src="product1.jpg" alt="商品1"> <p>商品1</p> <p>价格:100元</p> <form> <label for="quantity1">数量:</label> <input type="number" id="quantity1" min="1" value="1"> <button type="submit">更新</button> </form> <button class="remove">删除</button> </li> <li> <img src="product2.jpg" alt="商品2"> <p>商品2</p> <p>价格:200元</p> <form> <label for="quantity2">数量:</label> <input type="number" id="quantity2" min="1" value="1"> <button type="submit">更新</button> </form> <button class="remove">删除</button> </li> </ul> <p>总价:300元</p> <button id="checkout">结算</button> </main> <footer> <p>版权所有 © 2021</p> </footer> </body> </html>
这份 HTML 代码已经包含了一个基本的购物车页面的结构和样式,但是还需要一个 JavaScript 文件来添加一些交互和功能。以下是一个示例 JavaScript 文件,你可以在 script.js 文件中添加它:
```
// 获取所需的元素
const quantityInputs = document.querySelectorAll('input[type="number"]');
const updateButtons = document.querySelectorAll('form button[type="submit"]');
const removeButtons = document.querySelectorAll('.remove');
const checkoutButton = document.getElementById('checkout');
const totalPrice = document.querySelector('main p');
// 计算总价
function calculateTotalPrice() {
let total = 0;
quantityInputs.forEach((input, index) => {
const price = input.parentNode.querySelector('p:last-of-type').textContent.slice(3);
total += parseInt(price) * parseInt(input.value);
});
totalPrice.textContent = `总价:${total}元`;
}
// 更新数量
function updateQuantity(event) {
event.preventDefault();
const input = event.target.previousElementSibling;
const price = event.target.parentNode.querySelector('p:last-of-type').textContent.slice(3);
const subtotal = parseInt(price) * parseInt(input.value);
event.target.parentNode.parentNode.querySelector('p:last-of-type').textContent = `小计:${subtotal}元`;
calculateTotalPrice();
}
// 删除商品
function removeItem(event) {
event.target.parentNode.remove();
calculateTotalPrice();
}
// 点击更新按钮时更新数量
updateButtons.forEach(button => {
button.addEventListener('click', updateQuantity);
});
// 点击删除按钮时删除商品
removeButtons.forEach(button => {
button.addEventListener('click', removeItem);
});
// 点击结算按钮时弹出提示框
checkoutButton.addEventListener('click', () => {
alert('结算成功!');
});
```
这段代码包含了三个函数,分别用于更新数量、删除商品和计算总价。还有一些事件监听器,用于监听更新按钮、删除按钮和结算按钮的点击事件。在每个事件监听器中,都会调用对应的函数来实现相应的功能。最后,你需要将这个 JavaScript 文件链接到你的 HTML 文件中,就可以让页面具备交互和功能了。
相关推荐
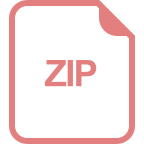
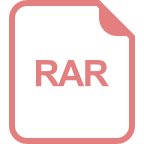
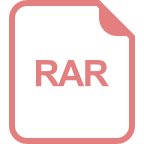






<!DOCTYPE html> <html> <head> <title>登录界面</title> <meta charset="utf-8" /> <title></title> </head> <style> input[type="text"], input[type="password"] { display: block; width: 5%; padding: 10px; margin-bottom: 20px; border-radius: 5px; border: 1px solid #ccc; font-size: 1.2em; } body{ background: url(image/java.jpg) top left; width: 10240px; height: 5120px; background-attachment: fixed; background-repeat: no-repeat; background-size: 100%; } 00 input[type="submit"] { background-color: Transparent; color: #abc1ee; padding: 10px 20px; border-radius: 5px; border: none; font-size: 1.2em; cursor: pointer; } a { text-decoration-line: none; color: #abc1ee; } </style> <body> Welcome to Login Login <input type="text" name="username" placeholder="username" id="username"> <input type="password" name="password" placeholder="password" id="password"> <input type="submit" value="Login" onclick="validate()"> Don't have account? Sign up <script> // 定义全局变量 var usernameInput = document.getElementById("username"); var passwordInput = document.getElementById("password"); var errorCount = 0; function validate() { // 定义局部变量 var username = usernameInput.value; var password = passwordInput.value; // switch...case语句 switch (username) { case "": alert("用户名不能为空"); errorCount++; break; case "admin": if (password === "admin") { alert("登录成功"); } else { alert("密码错误"); errorCount++; } break; default: alert("用户名不存在"); errorCount++; break; } // for...in语句 for (var i in username) { if (username[i] === " ") { alert("用户名不能包含空格"); errorCount++; break; } } // if语句 if (password === "") { alert("密码不能为空"); errorCount++; } // 调用函数 displayError(); if (errorCount >= 3) { alert("错误次数过多,请稍后再试"); window.location.reload(); } } function displayError() { // 获取元素 var container = document.querySelector(".container"); // 创建元素节点 var errorDiv = document.createElement("div"); errorDiv.innerHTML = "输入有误,请重新输入"; errorDiv.style.color = "red"; // 对子节点的操作 container.appendChild(errorDiv); setTimeout(function() { container.removeChild(errorDiv); }, 3000); } </script> </body> </html>对这个界面进行优化,添加标签






