<script setup> const getAnchorClothing = async (id) => { const res = await getAnchorClothingList(id) anchorClothingList.value = res.data.data console.log(res); } const getClothes = () => { getAnchorClothing(humanClothesList.value.id) // 设置服装 dialogHumanSetVisible.value = true } </script> <template> <div class="picSet"> <button @click="getClothes">数字人与画面设置</button> </div> <div class="imgShow"> <img :src=humanClothesList.back_url> </div> <el-dialog v-model="dialogHumanSetVisible" class="humanSet" :close-on-click-modal="false"> <div class="clothing" v-for=" item in anchorClothingList " :key="item.id" tabindex="1"> <img :src="item.image" @click="selectClothingImage(item)"> </div> <div class="right"> <el-button @click="dialogHumanSetVisible = false" class="cancelHandle">取消</el-button> <el-button @click="imageShowSubmit()" class="confirmHandle">确认</el-button> </div> </el-dialog> </template> vue3选择对话框中v-for循环的单个图片,然后点击确认后,所选择的图片会显示class="imgShow"下的img中,替换掉原来的图片,并且对话框隐藏,点击取消则无变化
时间: 2024-01-03 07:15:21 浏览: 99
根据你的描述,你需要实现以下功能:
1. 点击按钮时弹出选择对话框,其中包含多个服装图片;
2. 点击对话框中的某个服装图片后,将该图片显示在页面上的 `img` 标签中;
3. 点击对话框中的确认按钮后,将所选服装图片替换原来的图片,并隐藏对话框;
4. 点击对话框中的取消按钮后,不做任何变化。
可以按照以下步骤实现该功能:
1. 定义一个 `selectedClothing` 变量来保存当前所选的服装图片,初始值为 `null`;
2. 在对话框中使用 `v-for` 循环渲染服装图片,同时给每个图片绑定一个 `click` 事件,在点击某个图片时将 `selectedClothing` 变量更新为该图片的信息;
3. 在 `img` 标签中通过 `:src` 属性绑定 `selectedClothing` 变量,以便在所选服装图片改变时更新显示的图片;
4. 在确认按钮的 `click` 事件中将 `dialogHumanSetVisible` 变量设为 `false`,以隐藏对话框;
5. 在取消按钮的 `click` 事件中不做任何处理。
下面是完整的代码示例:
```html
<template>
<div class="picSet">
<button @click="dialogHumanSetVisible = true">数字人与画面设置</button>
</div>
<div class="imgShow">
<img :src="selectedClothing ? selectedClothing.image : humanClothesList.back_url">
</div>
<el-dialog v-model="dialogHumanSetVisible" class="humanSet" :close-on-click-modal="false">
<div class="clothing" v-for="item in anchorClothingList" :key="item.id" tabindex="1" @click="selectedClothing = item">
<img :src="item.image">
</div>
<div class="right">
<el-button @click="dialogHumanSetVisible = false" class="cancelHandle">取消</el-button>
<el-button @click="dialogHumanSetVisible = false; humanClothesList.back_url = selectedClothing ? selectedClothing.image : humanClothesList.back_url" class="confirmHandle">确认</el-button>
</div>
</el-dialog>
</template>
<script setup>
import { ref } from 'vue'
// 定义变量
const anchorClothingList = ref([])
const dialogHumanSetVisible = ref(false)
const selectedClothing = ref(null)
// 定义方法
const getAnchorClothing = async (id) => {
const res = await getAnchorClothingList(id)
anchorClothingList.value = res.data.data
console.log(res)
}
const getClothes = () => {
getAnchorClothing(humanClothesList.value.id)
dialogHumanSetVisible.value = true
}
const selectClothingImage = (item) => {
selectedClothing.value = item
}
const imageShowSubmit = () => {
humanClothesList.back_url = selectedClothing.value ? selectedClothing.value.image : humanClothesList.back_url
}
</script>
```
在确认按钮的 `click` 事件中,除了将 `dialogHumanSetVisible` 设为 `false`,还需要更新 `humanClothesList.back_url` 的值为所选服装图片的 `image` 属性,以便在确认后将其显示在页面上。
阅读全文
相关推荐
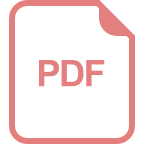
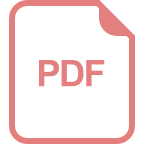
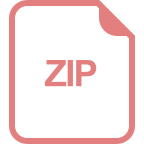










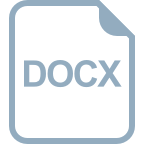
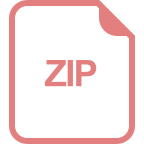
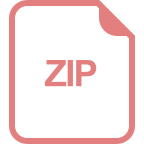


