error - found cycle in the listnode
时间: 2023-04-20 17:03:41 浏览: 128
这个错误提示意味着在链表中发现了循环。链表是一种数据结构,其中每个节点都包含一个值和一个指向下一个节点的指针。如果链表中存在循环,意味着其中一个节点的指针指向了链表中的一个之前的节点,导致链表形成了一个环。这通常是由于代码中的错误逻辑或指针错误引起的。需要检查代码并修复指针错误,以确保链表不会形成循环。
相关问题
#include <iostream> using namespace std; struct ListNode { int val; ListNode* next; ListNode(int x) : val(x), next(NULL) {} }; ListNode* mergeTwoLists(ListNode* l1, ListNode* l2) { ListNode* dummy = new ListNode(0); ListNode* cur = dummy; while (l1 && l2) { if (l1->val <= l2->val) { cur->next = l1; l1 = l1->next; } else { cur->next = l2; l2 = l2->next; } cur = cur->next; } if (l1) { cur->next = l1; } else { cur->next = l2; } return dummy->next; } int main() { ListNode* l1 = new ListNode(1); l1->next = new ListNode(3); l1->next->next = new ListNode(5); ListNode* l2 = new ListNode(2); l2->next = new ListNode(4); l2->next->next = new ListNode(6); ListNode* merged = mergeTwoLists(l1, l2); while (merged) { cout << merged->val << " "; merged = merged->next; } return 0; }将这段代码中的有序表改成可自己输入
好的,你可以参考以下代码,其中cin输入的数字需要自己输入,每输入一个数字按回车键确认,输入完毕后输入-1结束输入。
```cpp
#include <iostream>
using namespace std;
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(NULL) {}
};
ListNode* mergeTwoLists(ListNode* l1, ListNode* l2) {
ListNode* dummy = new ListNode(0);
ListNode* cur = dummy;
while (l1 && l2) {
if (l1->val <= l2->val) {
cur->next = l1;
l1 = l1->next;
} else {
cur->next = l2;
l2 = l2->next;
}
cur = cur->next;
}
if (l1) {
cur->next = l1;
} else {
cur->next = l2;
}
return dummy->next;
}
int main() {
ListNode* l1 = new ListNode(0);
ListNode* l2 = new ListNode(0);
ListNode* cur1 = l1;
ListNode* cur2 = l2;
int num;
cout << "Please enter the numbers for the first linked list: " << endl;
while (cin >> num && num != -1) {
cur1->next = new ListNode(num);
cur1 = cur1->next;
}
cout << "Please enter the numbers for the second linked list: " << endl;
while (cin >> num && num != -1) {
cur2->next = new ListNode(num);
cur2 = cur2->next;
}
ListNode* merged = mergeTwoLists(l1->next, l2->next);
while (merged) {
cout << merged->val << " ";
merged = merged->next;
}
return 0;
}
```
希望能够帮到你!
#include <iostream> using namespace std;// 定义单链表结构体 struct ListNode { int val; ListNode* next; ListNode(int x) : val(x), next(NULL) { } }; // 定义单链表基本操作函数 class LinkedList { public: // 合并两个有序链表 static ListNode* mergeTwoLists(ListNode* l1, ListNode* l2) { ListNode dummy(0); ListNode* tail = &dummy; while (l1 && l2) { if (l1->val < l2->val) { tail->next = l1; l1 = l1->next; } else { tail->next = l2; l2 = l2->next; } tail = tail->next; } tail->next = l1 ? l1 : l2; return dummy.next; } }; int main() {// 定义两个有序链表 ListNode* l1 = new ListNode(1); l1->next = new ListNode(2); l1->next->next = new ListNode(4); ListNode* l2 = new ListNode(1); l2->next = new ListNode(3); l2->next->next = new ListNode(4); // 合并两个有序链表 ListNode* mergedList = LinkedList::mergeTwoLists(l1, l2); // 输出结果 while (mergedList) { cout << mergedList->val << " "; mergedList = mergedList->next; } cout << endl; return 0; }详细注释
这段代码实现了一个合并两个有序链表的功能,使用了单链表结构体和单链表基本操作函数。下面是详细注释:
```c++
#include <iostream>
using namespace std;
// 定义单链表结构体
struct ListNode {
int val; // 存储节点的值
ListNode* next; // 指向下一个节点的指针
// 构造函数
ListNode(int x) : val(x), next(NULL) { }
};
// 定义单链表基本操作函数
class LinkedList {
public:
// 合并两个有序链表
static ListNode* mergeTwoLists(ListNode* l1, ListNode* l2) {
ListNode dummy(0); // 定义虚拟头结点
ListNode* tail = &dummy; // 定义尾指针,指向虚拟头结点
while (l1 && l2) { // 遍历两个链表
if (l1->val < l2->val) { // 如果l1的节点值小于l2的节点值
tail->next = l1; // 将l1的节点加入合并后的链表中
l1 = l1->next; // l1指针后移
}
else { // 否则
tail->next = l2; // 将l2的节点加入合并后的链表中
l2 = l2->next; // l2指针后移
}
tail = tail->next; // 尾指针后移
}
tail->next = l1 ? l1 : l2; // 将剩余的节点加入合并后的链表中
return dummy.next; // 返回合并后的链表头结点
}
};
int main() {
// 定义两个有序链表
ListNode* l1 = new ListNode(1);
l1->next = new ListNode(2);
l1->next->next = new ListNode(4);
ListNode* l2 = new ListNode(1);
l2->next = new ListNode(3);
l2->next->next = new ListNode(4);
// 合并两个有序链表
ListNode* mergedList = LinkedList::mergeTwoLists(l1, l2);
// 输出结果
while (mergedList) {
cout << mergedList->val << " ";
mergedList = mergedList->next;
}
cout << endl;
return 0;
}
```
代码中首先定义了一个单链表结构体 `ListNode`,包含一个 `val` 成员变量和一个指向下一个节点的指针 `next`。然后定义了一个单链表基本操作函数 `LinkedList`,其中的 `mergeTwoLists` 函数用于合并两个有序链表。该函数使用了一个虚拟头结点 `dummy` 和一个尾指针 `tail`,遍历两个链表并将节点依次加入合并后的链表中。最后返回合并后的链表头结点。
在 `main` 函数中,定义了两个有序链表 `l1` 和 `l2`,调用 `LinkedList` 的 `mergeTwoLists` 函数将两个链表合并,并将合并后的链表输出到控制台。
阅读全文
相关推荐
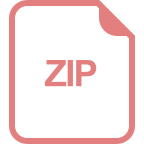
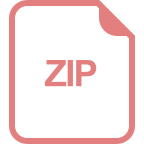












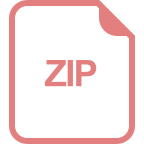