帮忙写一个paypal smart button pay的详细例子,结合js和.net web api
时间: 2024-12-11 14:43:04 浏览: 8
PayPal Smart Button是一种简单的JavaScript支付组件,它允许开发者轻松地将PayPal付款集成到网站上。下面是一个基本的例子,展示如何在.NET Web API项目中创建一个PayPal Smart Button,并通过JS在前端触发支付。
首先,在前端HTML中添加Smart Button:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<title>Pay with PayPal</title>
<script defer src="https://www.paypal.com/sdk/js?client-id=YOUR_CLIENT_ID"></script>
<style>
.paypal-button {
margin-bottom: 10px;
}
</style>
</head>
<body>
<div id="paypal-button-container">
<button class="paypal-button" data-gateway="paypal" data-commit="true">Pay with PayPal</button>
</div>
<script>
paypal.Buttons({
createOrder: function(data, actions) {
return actions.order.create({
purchase_units: [{
amount: { currency_code: 'USD', value: '10.00' } // 替换为你实际的商品价格
}]
});
},
onApprove: async function(orderId) {
try {
const response = await fetch('/api/paypal/order/' + orderId, {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({ orderId })
});
if (response.ok) {
alert('Payment successful!');
} else {
alert('Payment failed. Please try again.');
}
} catch (error) {
console.error(error);
}
}
}).render('#paypal-button-container');
</script>
</body>
</html>
```
这里,你需要替换`YOUR_CLIENT_ID`为你的PayPal Developer账户中应用的Client ID。`createOrder`函数用于初始化订单,`onApprove`将在用户确认支付后执行,并向后端发送订单ID。
然后,在`.NET Core Web API`项目的控制器中处理支付请求:
```csharp
using Microsoft.AspNetCore.Mvc;
using YourProjectNamespace; // 自定义一个包含PayPal响应模型的命名空间
[ApiController]
[Route("[controller]")]
public class PayPalController : ControllerBase
{
[HttpPost("order/{orderId}")]
public IActionResult PayWithPayPal([FromBody] string orderId)
{
// 这里你通常需要调用PayPal SDK或API来验证订单并完成交易
// 假设你有一个处理PayPal回调的函数,例如 CallPayPalApi()
var result = CallPayPalApi(orderId);
if (result.Success)
{
return Ok(new { success = true, message = "Payment completed." });
}
else
{
return BadRequest(new { error = result.ErrorMessage, status = result.ErrorStatusCode });
}
}
private class PaymentResult
{
public bool Success { get; set; }
public string ErrorMessage { get; set; }
public int ErrorStatusCode { get; set; }
}
private static PaymentResult CallPayPalApi(string orderId)
{
// 实际的PayPal API调用逻辑,此处仅作示例
// 连接到PayPal,验证订单状态,如果成功则返回Success,错误信息等
// ...
}
}
```
确保在`CallPayPalApi`方法中正确地连接到PayPal、验证订单,并处理相应的支付结果。
阅读全文
相关推荐
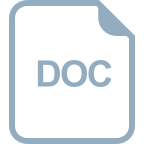
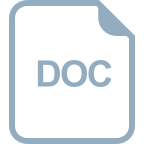
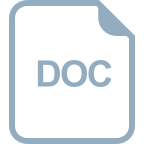
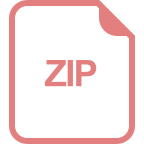
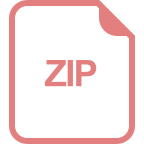
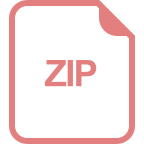
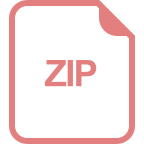
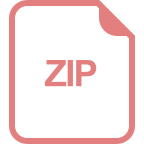
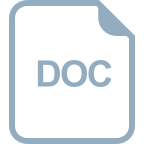
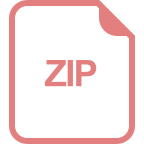
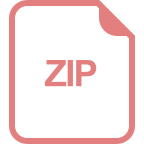
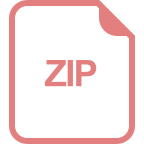
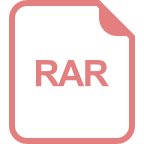
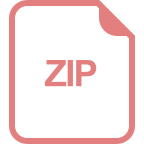
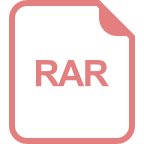
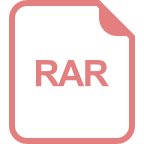
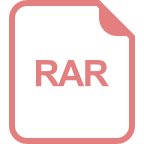
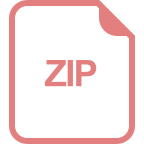
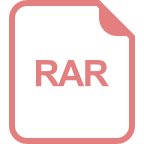