写一个兔子拔萝卜吃萝卜的C++程序,它包含两个类:兔子类和萝卜类。萝卜类有一个静态公有的数量变量,并且使用vector容器来存储。兔子类嵌套了萝卜数量的容器,当程序输入了拔了几个萝卜吃了几个萝卜时,它会把吃了几个萝卜从萝卜数量容器中减去,最后输出还剩下几个萝卜或者萝卜不够吃。
时间: 2023-09-23 20:06:06 浏览: 221
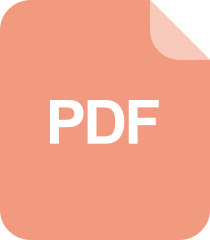
关于C++的类
### 回答1:
下面是一个写兔子拔萝卜吃萝卜的程序:
```
#include<iostream>
#include<vector>
using namespace std;
class Carrot
{
public:
static int quantity;
};
int Carrot::quantity = 0;
class Rabbit
{
public:
vector<Carrot> carrots;
void pullCarrots(int n)
{
for (int i = 0; i < n; i++)
{
carrots.push_back(Carrot());
}
Carrot::quantity += n;
}
void eatCarrots(int m)
{
if (m > Carrot::quantity)
{
cout << "萝卜不够吃" << endl;
}
else
{
Carrot::quantity -= m;
cout << "还剩下" << Carrot::quantity << "个萝卜" << endl;
}
}
};
int main()
{
Rabbit rabbit;
int n, m;
cout << "请输入拔了几个萝卜:";
cin >> n;
rabbit.pullCarrots(n);
cout << "请输入吃了几个萝卜:";
cin >> m;
rabbit.eatCarrots(m);
return 0;
}
```
### 回答2:
下面是一个满足题目要求的C++程序:
```cpp
#include <iostream>
#include <vector>
using namespace std;
class 萝卜类 {
public:
static int 数量;
};
int 萝卜类::数量 = 0;
class 兔子类 {
public:
兔子类() {
萝卜数量.reserve(10); // 假设最多有10个萝卜
}
void 拔萝卜(int 拔掉的数量) {
if (萝卜类::数量 < 拔掉的数量) {
cout << "萝卜不够吃" << endl;
} else {
for (int i = 0; i < 拔掉的数量; i++) {
萝卜类::数量--;
萝卜数量.pop_back();
}
cout << "还剩下" << 萝卜类::数量 << "个萝卜" << endl;
}
}
private:
vector<int> 萝卜数量;
};
int main() {
萝卜类 萝卜;
兔子类 兔子;
萝卜.数量 = 5;
兔子.拔萝卜(3);
兔子.拔萝卜(2);
return 0;
}
```
程序中首先定义了一个萝卜类,它含有一个静态公有的数量变量,用来存储萝卜的总数量。然后定义了兔子类,它嵌套了一个私有的萝卜数量容器,使用vector来实现。兔子类中有一个拔萝卜的方法,该方法接受一个参数表示要拔掉的萝卜数量。如果萝卜数量不足,则输出"萝卜不够吃";否则从萝卜数量容器和萝卜总数量中减去相应数量的萝卜,并输出剩余的萝卜数量。在主函数中,初始化了5个萝卜,并调用兔子类的拔萝卜方法拔掉3个和2个萝卜,最后输出剩余的萝卜数量。
### 回答3:
以下是一个满足题目要求的 C++ 程序示例:
```cpp
#include <iostream>
#include <vector>
using namespace std;
class Carrot {
public:
static int count; // 静态公有的数量变量
};
class Rabbit {
private:
vector<int> carrots; // 萝卜数量的容器
public:
void pickCarrots(int num) { // 拔了几个萝卜
for (int i = 0; i < num; i++) {
if (Carrot::count > 0) {
Carrot::count--;
carrots.push_back(i); // 将拔的萝卜放入兔子的容器中
} else {
cout << "萝卜不够吃" << endl;
return;
}
}
}
void eatCarrots(int num) { // 吃了几个萝卜
if (num > carrots.size()) {
cout << "还剩下 " << carrots.size() << " 个萝卜" << endl;
} else {
for (int i = 0; i < num; i++) {
carrots.pop_back(); // 从兔子的容器中减去吃的萝卜
}
cout << "还剩下 " << carrots.size() << " 个萝卜" << endl;
}
}
};
int Carrot::count = 10; // 初始化萝卜数量为10个
int main() {
Rabbit rabbit;
int pick, eat;
cout << "请输入拔了几个萝卜:";
cin >> pick;
cout << "请输入吃了几个萝卜:";
cin >> eat;
rabbit.pickCarrots(pick);
rabbit.eatCarrots(eat);
return 0;
}
```
该程序中,`Carrot` 类负责记录萝卜的数量,使用静态公有的 `count` 变量来实现。`Rabbit` 类则负责处理兔子拔萝卜和吃萝卜的逻辑。通过 `vector<int>` 容器存储兔子拔的萝卜,其中的 `pickCarrots` 方法用于拔萝卜,`eatCarrots` 方法用于吃萝卜。程序主函数中输入要拔和吃的萝卜数量后,调用相应的方法进行操作,并输出剩余的萝卜数量或提示萝卜不够吃。
阅读全文
相关推荐





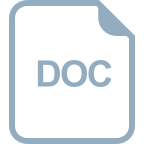
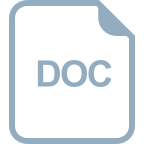
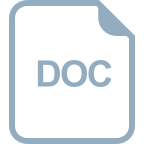
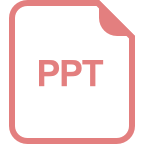