在触摸屏上点三点显示一个实心三角形C语言程序
时间: 2024-02-28 21:56:18 浏览: 127
下面是一个简单的C语言程序,实现了在触摸屏上点三点并显示一个实心三角形的功能。该程序使用了OpenGL图形库来绘制三角形,并使用了Linux系统的触摸屏API获取用户的点击点坐标。
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#include <linux/input.h>
#include <fcntl.h>
#include <unistd.h>
#include <GL/glut.h>
// 触摸屏设备文件
#define TOUCHSCREEN_DEVICE "/dev/input/event0"
// 触摸屏分辨率
#define TOUCHSCREEN_WIDTH 800
#define TOUCHSCREEN_HEIGHT 480
// 三角形的三个顶点坐标
GLfloat vertices[3][2] = {{0.0, 0.0}, {0.0, 0.0}, {0.0, 0.0}};
// 三角形填充颜色
GLfloat color[3] = {1.0, 0.0, 0.0};
// 三角形是否可见
GLboolean visible = GL_FALSE;
// 初始化OpenGL窗口
void init(void)
{
glClearColor(0.0, 0.0, 0.0, 0.0);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
gluOrtho2D(0.0, TOUCHSCREEN_WIDTH, 0.0, TOUCHSCREEN_HEIGHT);
}
// 绘制三角形
void display(void)
{
glClear(GL_COLOR_BUFFER_BIT);
if (visible) {
glColor3fv(color);
glBegin(GL_TRIANGLES);
glVertex2fv(vertices[0]);
glVertex2fv(vertices[1]);
glVertex2fv(vertices[2]);
glEnd();
}
glFlush();
}
// 处理触摸屏事件
void handle_touchscreen_event(struct input_event *ev)
{
static int count = 0;
static int x, y;
if (ev->type == EV_ABS) {
switch (ev->code) {
case ABS_X:
x = ev->value;
break;
case ABS_Y:
y = ev->value;
break;
}
} else if (ev->type == EV_KEY && ev->code == BTN_TOUCH) {
if (ev->value == 1) {
// 用户点击屏幕,记录点击点坐标并绘制三角形
vertices[count][0] = x;
vertices[count][1] = TOUCHSCREEN_HEIGHT - y;
count++;
if (count == 3) {
visible = GL_TRUE;
glutPostRedisplay();
count = 0;
}
}
}
}
// 消息循环函数
void event_loop(void)
{
int fd;
struct input_event ev;
// 打开触摸屏设备文件
fd = open(TOUCHSCREEN_DEVICE, O_RDONLY);
if (fd < 0) {
perror("open");
exit(EXIT_FAILURE);
}
// 监听触摸屏事件并处理
while (1) {
read(fd, &ev, sizeof(ev));
handle_touchscreen_event(&ev);
}
}
int main(int argc, char **argv)
{
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_SINGLE | GLUT_RGB);
glutInitWindowSize(TOUCHSCREEN_WIDTH, TOUCHSCREEN_HEIGHT);
glutCreateWindow("Triangle");
init();
glutDisplayFunc(display);
glutFullScreen();
event_loop();
return 0;
}
```
注意,该程序是一个不完整的示例程序,仅演示了如何使用OpenGL和触摸屏API实现在触摸屏上点三点并显示一个实心三角形的功能。实际应用中,需要根据具体的需求进行修改和完善。
阅读全文
相关推荐
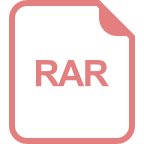















