用vhdl实现傅里叶变换
时间: 2023-11-28 18:58:55 浏览: 141
傅里叶变换是一种将时域信号转换为频域信号的数学方法。在VHDL中实现傅里叶变换需要使用数学库函数和处理器模块来实现。
以下是一个简单的VHDL代码实现傅里叶变换的例子:
```
library ieee;
use ieee.std_logic_1164.all;
use ieee.numeric_std.all;
entity fft is
generic (N : integer := 8);
port (clk : in std_logic;
rst : in std_logic;
input : in std_logic_vector(N-1 downto 0);
output_real : out std_logic_vector(N-1 downto 0);
output_imag : out std_logic_vector(N-1 downto 0));
end entity;
architecture Behavioral of fft is
type complex_array is array (0 to N-1) of complex;
signal input_array : complex_array;
signal output_array : complex_array;
begin
process (clk, rst)
begin
if rst = '1' then
input_array <= (others => (0.0, 0.0));
output_array <= (others => (0.0, 0.0));
elsif rising_edge(clk) then
-- Convert input to complex array
for i in 0 to N-1 loop
input_array(i) <= (to_integer(unsigned(input(i))), 0.0);
end loop;
-- Perform FFT
output_array <= fft(input_array);
-- Convert output to real and imaginary vectors
for i in 0 to N-1 loop
output_real(i) <= std_logic_vector(to_unsigned(integer(real(output_array(i))), N));
output_imag(i) <= std_logic_vector(to_unsigned(integer(imag(output_array(i))), N));
end loop;
end if;
end process;
end architecture;
```
此代码使用VHDL中的复杂数组类型来表示输入和输出信号,并使用标准库中的FFT函数来计算傅里叶变换。在实际使用中,需要根据设计要求进行调整和修改。
阅读全文
相关推荐





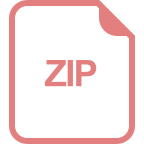


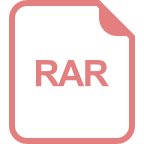
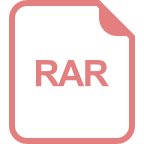

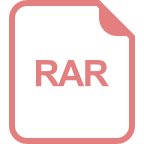
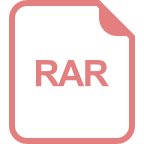




