C++判断字符串非空empty()
时间: 2023-09-18 19:13:56 浏览: 49
在C++中,可以使用字符串的成员函数 `empty()` 来判断字符串是否为空,当字符串为空时,返回值为 `true`,否则返回值为 `false`。可以使用以下代码判断:
```cpp
if (my_string.empty()) {
// 空字符串
} else {
// 非空字符串
}
```
也可以通过字符串的长度来判断字符串是否为空,当字符串的长度为0时,即为空字符串。可以使用以下代码判断:
```cpp
if (my_string.length() == 0) {
// 空字符串
} else {
// 非空字符串
}
```
其中,`length()` 是字符串的成员函数,用于获取字符串的长度。
相关问题
C++ 判断string非空
可以使用字符串的 `empty()` 函数来判断字符串是否为空。具体实现如下:
```cpp
#include <iostream>
#include <string>
using namespace std;
int main() {
string str = "Hello World!";
if (str.empty()) {
cout << "字符串为空" << endl;
} else {
cout << "字符串不为空" << endl;
}
return 0;
}
```
如果字符串为空,输出结果为:字符串为空;否则,输出结果为:字符串不为空。
c++判断string不为空
在C语言中,可以通过以下两种方法来判断一个字符串是否为空:
方法一:使用字符串的长度判断。首先,我们需要删除字符串中的所有空格,然后判断处理后的字符串是否为空。如果为空,则表示原始字符串为空;否则,表示原始字符串不为空。可以使用以下代码实现:
```c
int32_t has_only_spaces(string &str) {
string tmpStr = str;
u_int32_t length = tmpStr.length();
// 将字符串中的所有空格删除
while (length > 0) {
length--;
string::size_type index = tmpStr.find(' ');
if (index != string::npos) {
tmpStr.erase(index, 1);
}
}
// 判断字符串是否为空
if (tmpStr.empty()) {
return -1; // 空
} else {
return 0; // 非空
}
}
```
方法二:使用字符串类的成员函数empty()。可以直接调用字符串的empty()函数来判断字符串是否为空。如果返回true,则表示字符串为空;否则,表示字符串不为空。可以使用以下代码实现:
```c
string str;
if (str.empty()) {
// 字符串为空
} else {
// 字符串不为空
}
```
无论使用哪种方法,你都可以判断一个字符串是否为空。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* [c++判断string是否为空](https://blog.csdn.net/weixin_42129772/article/details/122566835)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
- *2* *3* [c++ string类判断字符串是否为空](https://blog.csdn.net/weixin_48010724/article/details/124661970)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
相关推荐







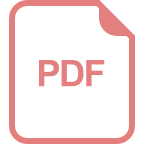
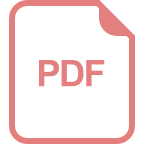
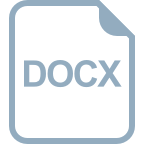
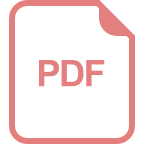