汇编实现kmp
时间: 2023-07-19 13:09:50 浏览: 57
汇编语言实现KMP算法的步骤如下:
1. 定义输入字符串和模式串的内存空间。
2. 将输入字符串和模式串加载到内存中。
3. 初始化next数组。
4. 依次遍历输入字符串,对每个字符进行匹配。
5. 当匹配失败时,根据next数组移动模式串的指针。
6. 当匹配成功时,输出匹配位置,并移动模式串的指针。
下面是一段使用汇编语言实现KMP算法的代码:
```
; 定义输入字符串和模式串的内存空间
input_string db 'ababcabcacbab',0
pattern_string db 'abcac',0
; 定义next数组
next_array dw 0,0,0,0,0
; 初始化next数组
mov cx, 4
mov si, 0
mov di, 2
mov ax, 1
next_loop:
cmp si, 0
je next_skip
cmp byte[pattern_string+si], byte[pattern_string+di-1]
je next_match
mov si, word[next_array+si*2-2]
jmp next_loop
next_match:
mov word[next_array+di*2-2], ax
inc si
inc di
mov ax, di
jmp next_loop
next_skip:
mov word[next_array], 0
mov word[next_array+2], 0
inc di
cmp di, cx
jge next_done
mov ax, di
jmp next_loop
next_done:
; 遍历输入字符串,进行匹配
mov si, 0
mov di, 0
mov cx, 0
input_loop:
cmp byte[input_string+si+cx], 0
je input_done
cmp byte[input_string+si+cx], byte[pattern_string+di]
jne input_mismatch
inc di
cmp byte[pattern_string+di], 0
je input_match
inc cx
jmp input_loop
input_match:
mov ax, si
add ax, cx
sub ax, di
mov dx, ax
mov ah, 9
lea dx, [input_string+dx]
int 21h
inc cx
mov di, word[next_array+di*2-2]
jmp input_loop
input_mismatch:
cmp di, 0
je input_next
mov di, word[next_array+di*2-2]
jmp input_mismatch
input_next:
inc si
jmp input_loop
input_done:
```
以上代码实现了KMP算法的功能,你可以根据需要进行修改和优化。
相关推荐
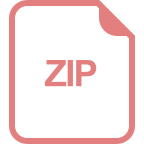














