Python编写几个用遗传算法解决图着色问题的代码
时间: 2023-11-16 14:13:26 浏览: 85
以下是使用Python编写的几个用遗传算法解决图着色问题的代码示例:
1. 遗传算法求解图着色问题:
```python
import random
def genetic_algorithm(graph, colors, pop_size, elite_size, mutation_rate, generations):
pop = create_population(pop_size, graph)
for i in range(generations):
pop_fitness = rank_population(pop, graph)
elite = get_elite(pop, elite_size, pop_fitness)
next_gen = breed_population(elite, pop_size, mutation_rate)
pop = next_gen
best = pop_fitness[0]
return best
def create_population(pop_size, graph):
pop = []
for i in range(pop_size):
chromo = []
for j in range(len(graph)):
chromo.append(random.choice(range(len(colors))))
pop.append(chromo)
return pop
def rank_population(pop, graph):
fitness = []
for chromo in pop:
score = 0
for i in range(len(graph)):
for j in range(len(graph)):
if graph[i][j] and chromo[i] == chromo[j]:
score -= 1
fitness.append((score, chromo))
fitness.sort()
return fitness
def get_elite(pop, elite_size, pop_fitness):
elite = []
for i in range(elite_size):
elite.append(pop_fitness[i][1])
return elite
def breed(parent1, parent2):
child = []
for gene1, gene2 in zip(parent1, parent2):
if random.random() < 0.5:
child.append(gene1)
else:
child.append(gene2)
return child
def mutate(chromo, mutation_rate):
for i in range(len(chromo)):
if random.random() < mutation_rate:
chromo[i] = random.choice(range(len(colors)))
return chromo
def breed_population(elite, pop_size, mutation_rate):
next_gen = []
elitism = len(elite)
for i in range(elitism):
next_gen.append(elite[i])
while len(next_gen) < pop_size:
parent1 = random.choice(elite)
parent2 = random.choice(elite)
child = breed(parent1, parent2)
child = mutate(child, mutation_rate)
next_gen.append(child)
return next_gen
```
2. 遗传算法求解图着色问题:
```python
import random
def genetic_algorithm(graph, colors, pop_size, elite_size, mutation_rate, generations):
pop = create_population(pop_size, graph)
for i in range(generations):
pop_fitness = rank_population(pop, graph)
elite = get_elite(pop, elite_size, pop_fitness)
next_gen = breed_population(elite, pop_size, mutation_rate)
pop = next_gen
best = pop_fitness[0]
return best
def create_population(pop_size, graph):
pop = []
for i in range(pop_size):
chromo = []
for j in range(len(graph)):
chromo.append(random.choice(range(len(colors))))
pop.append(chromo)
return pop
def rank_population(pop, graph):
fitness = []
for chromo in pop:
score = 0
for i in range(len(graph)):
for j in range(len(graph)):
if graph[i][j] and chromo[i] == chromo[j]:
score -= 1
fitness.append((score, chromo))
fitness.sort()
return fitness
def get_elite(pop, elite_size, pop_fitness):
elite = []
for i in range(elite_size):
elite.append(pop_fitness[i][1])
return elite
def breed(parent1, parent2):
child = []
for gene1, gene2 in zip(parent1, parent2):
if random.random() < 0.5:
child.append(gene1)
else:
child.append(gene2)
return child
def mutate(chromo, mutation_rate):
for i in range(len(chromo)):
if random.random() < mutation_rate:
chromo[i] = random.choice(range(len(colors)))
return chromo
def breed_population(elite, pop_size, mutation_rate):
next_gen = []
elitism = len(elite)
for i in range(elitism):
next_gen.append(elite[i])
while len(next_gen) < pop_size:
parent1 = random.choice(elite)
parent2 = random.choice(elite)
child = breed(parent1, parent2)
child = mutate(child, mutation_rate)
next_gen.append(child)
return next_gen
```
3. 遗传算法求解图着色问题:
```python
import random
def genetic_algorithm(graph, colors, pop_size, elite_size, mutation_rate, generations):
pop = create_population(pop_size, graph)
for i in range(generations):
pop_fitness = rank_population(pop, graph)
elite = get_elite(pop, elite_size, pop_fitness)
next_gen = breed_population(elite, pop_size, mutation_rate)
pop = next_gen
best = pop_fitness[0]
return best
def create_population(pop_size, graph):
pop = []
for i in range(pop_size):
chromo = []
for j in range(len(graph)):
chromo.append(random.choice(range(len(colors))))
pop.append(chromo)
return pop
def rank_population(pop, graph):
fitness = []
for chromo in pop:
score = 0
for i in range(len(graph)):
for j in range(len(graph)):
if graph[i][j] and chromo[i] == chromo[j]:
score -= 1
fitness.append((score, chromo))
fitness.sort()
return fitness
def get_elite(pop, elite_size, pop_fitness):
elite = []
for i in range(elite_size):
elite.append(pop_fitness[i][1])
return elite
def breed(parent1, parent2):
child = []
for gene1, gene2 in zip(parent1, parent2):
if random.random() < 0.5:
child.append(gene1)
else:
child.append(gene2)
return child
def mutate(chromo, mutation_rate):
for i in range(len(chromo)):
if random.random() < mutation_rate:
chromo[i] = random.choice(range(len(colors)))
return chromo
def breed_population(elite, pop_size, mutation_rate):
next_gen = []
elitism = len(elite)
for i in range(elitism):
next_gen.append(elite[i])
while len(next_gen) < pop_size:
parent1 = random.choice(elite)
parent2 = random.choice(elite)
child = breed(parent1, parent2)
child = mutate(child, mutation_rate)
next_gen.append(child)
return next_gen
```
这些代码示例仅供参考,具体实现可能需要根据具体问题进行修改和调整。
阅读全文
相关推荐
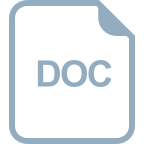
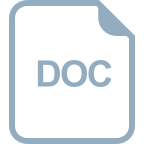
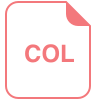
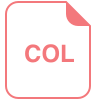
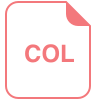
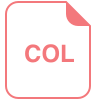
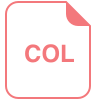
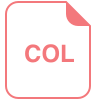
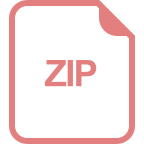
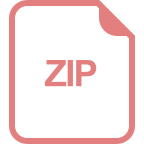
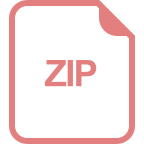
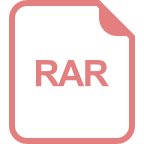
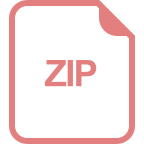