java selenium 判断元素是否有指定css样式
时间: 2023-10-07 22:05:14 浏览: 57
要判断元素是否具有指定的CSS样式,您可以使用Java中的Selenium WebDriver和ExpectedConditions类的`attributeContains`方法。以下是一个示例代码:
```java
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
public class ElementCSSStyleCheck {
public static void main(String[] args) {
// 设置驱动程序路径
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
// 初始化ChromeDriver实例
WebDriver driver = new ChromeDriver();
// 打开网页
driver.get("https://example.com");
// 等待元素加载完成
WebDriverWait wait = new WebDriverWait(driver, 10);
WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.cssSelector("your-selector")));
// 获取元素的CSS样式
String cssValue = element.getCssValue("your-css-property");
// 判断CSS样式是否包含指定的值
if (cssValue.contains("your-value")) {
System.out.println("Element has the specified CSS style.");
} else {
System.out.println("Element does not have the specified CSS style.");
}
// 关闭浏览器
driver.quit();
}
}
```
请将代码中的`"path/to/chromedriver"`替换为您本地ChromeDriver的路径,`"https://example.com"`替换为您要访问的网页URL,`"your-selector"`替换为您要查找的元素的CSS选择器,`"your-css-property"`替换为您要检查的CSS属性名称,`"your-value"`替换为您要检查的CSS属性值。
相关推荐
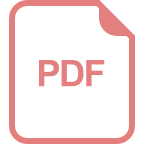
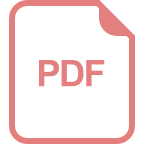














