QT使用gpu将RAW图转为RGB图,代码尽可能详细点
时间: 2023-06-12 13:06:59 浏览: 194
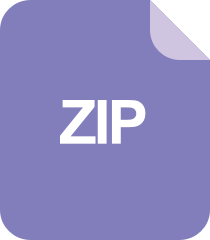
Qt对图片进行各种操作的开源代码
下面是一个简单的示例代码,使用CUDA加速将RAW图像转换为RGB图像。这个示例代码使用了NVIDIA CUDA库和OpenCV库,需要先安装它们。
```
#include <cuda_runtime.h>
#include <opencv2/opencv.hpp>
using namespace cv;
__global__ void raw_to_rgb_kernel(unsigned char* raw_data, unsigned char* rgb_data, int width, int height)
{
int x = blockIdx.x * blockDim.x + threadIdx.x;
int y = blockIdx.y * blockDim.y + threadIdx.y;
if (x >= width || y >= height) {
return;
}
int index = y * width + x;
int rgb_index = index * 3;
int raw_index = index * 2;
rgb_data[rgb_index] = raw_data[raw_index];
rgb_data[rgb_index + 1] = (raw_data[raw_index] + raw_data[raw_index + 1]) / 2;
rgb_data[rgb_index + 2] = raw_data[raw_index + 1];
}
void raw_to_rgb_gpu(unsigned char* raw_data, unsigned char* rgb_data, int width, int height)
{
const int block_size = 16;
dim3 dim_block(block_size, block_size);
dim3 dim_grid((width + block_size - 1) / block_size, (height + block_size - 1) / block_size);
raw_to_rgb_kernel<<<dim_grid, dim_block>>>(raw_data, rgb_data, width, height);
cudaDeviceSynchronize();
}
int main()
{
// Load raw data
Mat raw_image = imread("raw_image.raw", IMREAD_UNCHANGED);
if (raw_image.empty()) {
std::cerr << "Failed to load raw image" << std::endl;
return -1;
}
// Create RGB image
Mat rgb_image(raw_image.rows, raw_image.cols, CV_8UC3);
// Convert raw image to RGB using GPU
raw_to_rgb_gpu(raw_image.data, rgb_image.data, raw_image.cols, raw_image.rows);
// Save RGB image
imwrite("rgb_image.jpg", rgb_image);
return 0;
}
```
在这个示例代码中,`raw_to_rgb_kernel`是一个CUDA核函数,用于将RAW图像转换为RGB图像。它使用了CUDA的并行计算能力,可以显著提高转换速度。`raw_to_rgb_gpu`函数是一个CPU函数,用于在GPU上执行核函数。最后,我们加载RAW图像,将其转换为RGB图像,并将其保存为JPEG文件。
阅读全文
相关推荐
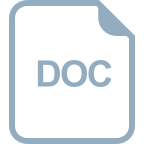
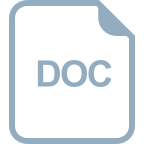




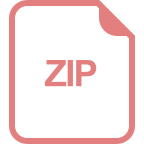
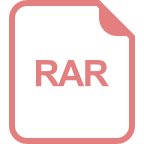
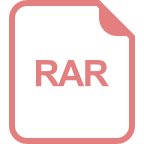
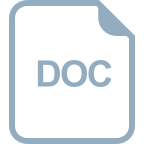





