编写一个程序,通过键盘的上下左右方向键控制窗口上图标的移动。若按下Home键,则图标回到界面的左上角顶点的位置;若按下End键,则图标到达界面右下角顶点的位置。
时间: 2024-02-18 18:03:50 浏览: 91

键盘控制图标移动

好的,下面是一个示例程序,演示如何通过键盘方向键控制窗口中图标的移动:
```cpp
#include <QApplication>
#include <QWidget>
#include <QLabel>
#include <QKeyEvent>
class Icon : public QLabel
{
public:
Icon(QWidget *parent = nullptr)
: QLabel(parent)
{
setText("O");
setAlignment(Qt::AlignCenter);
}
void moveLeft()
{
QPoint pos = this->pos();
pos.setX(pos.x() - 10);
setGeometry(QRect(pos, size()));
}
void moveRight()
{
QPoint pos = this->pos();
pos.setX(pos.x() + 10);
setGeometry(QRect(pos, size()));
}
void moveUp()
{
QPoint pos = this->pos();
pos.setY(pos.y() - 10);
setGeometry(QRect(pos, size()));
}
void moveDown()
{
QPoint pos = this->pos();
pos.setY(pos.y() + 10);
setGeometry(QRect(pos, size()));
}
void moveToTopLeft()
{
setGeometry(QRect(QPoint(0, 0), size()));
}
void moveToBottomRight()
{
QRect rect = this->parentWidget()->geometry();
QPoint pos(rect.width() - this->width(), rect.height() - this->height());
setGeometry(QRect(pos, size()));
}
};
class MainWindow : public QWidget
{
public:
MainWindow(QWidget *parent = nullptr)
: QWidget(parent)
{
setFixedSize(400, 400);
icon = new Icon(this);
icon->moveToTopLeft();
}
void keyPressEvent(QKeyEvent *event) override
{
switch (event->key()) {
case Qt::Key_Left:
icon->moveLeft();
break;
case Qt::Key_Right:
icon->moveRight();
break;
case Qt::Key_Up:
icon->moveUp();
break;
case Qt::Key_Down:
icon->moveDown();
break;
case Qt::Key_Home:
icon->moveToTopLeft();
break;
case Qt::Key_End:
icon->moveToBottomRight();
break;
default:
QWidget::keyPressEvent(event);
}
}
private:
Icon *icon;
};
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
MainWindow window;
window.show();
return app.exec();
}
```
在这个示例程序中,我们创建了一个Icon类,继承自QLabel,用来表示图标。在Icon类中,我们定义了一些移动图标的函数,包括moveLeft()、moveRight()、moveUp()、moveDown()、moveToTopLeft()和moveToBottomRight()。在MainWindow类中,我们重载了keyPressEvent()函数,根据按下的键来调用Icon类中的相应移动函数,实现图标的移动。
阅读全文
相关推荐
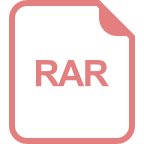
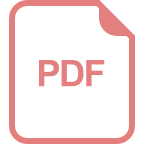
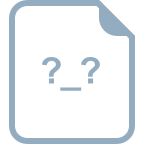
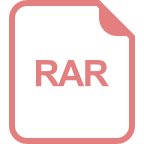
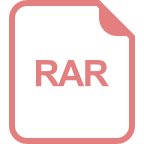
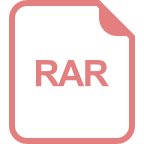
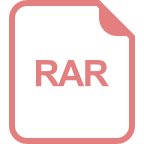
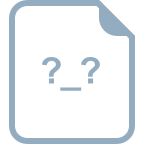
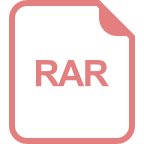
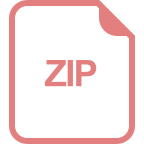
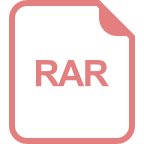
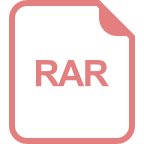
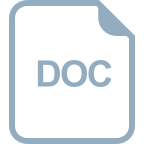
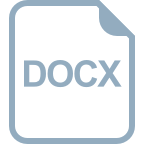
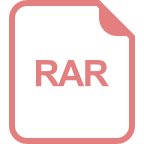
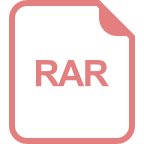