Performing an update on the path '_id' would modify the immutable field '_id'
时间: 2024-06-06 12:11:12 浏览: 182
As an AI language model, I cannot speculate on the specific context in which this message was encountered. However, in general, this error message indicates that an attempt was made to modify an immutable field (which cannot be changed) using the update command on a specific path that includes that field. This is not allowed because the field is marked as immutable, and therefore any attempt to modify it will result in an error. Possible solutions could include changing the field to mutable, or modifying the update path to exclude the immutable field.
相关问题
-- Looking for pthread.h - found -- Performing Test CMAKE_HAVE_LIBC_PTHREAD -- Performing Test CMAKE_HAVE_LIBC_PTHREAD - Failed -- Looking for pthread_create in pthreads -- Looking for pthread_create in pthreads - not found -- Looking for pthread_create in pthread -- Looking for pthread_create in pthread - found -- Found Threads: TRUE -- Looking for gethrtime -- Looking for gethrtime - not found -- Looking for socketpair -- Looking for socketpair - found -- Looking for eventfd -- Looking for eventfd - found -- Looking for pipe这是cmake的还是configure的
这是CMake的输出信息,其中包含了一些检查结果。CMake是一个跨平台的编译工具,用于自动生成Makefile文件,以便编译源代码。CMake配置文件通常命名为CMakeLists.txt。
在你的输出中,CMake正在检查一些系统库和头文件是否存在,并记录检查结果。例如,它正在检查pthread.h头文件是否存在,并在成功找到时打印“found”消息。它还在检查pthread库是否存在,并在找到时打印“found”消息。
这些检查是为了确定系统是否满足构建所需的依赖关系。如果有任何依赖项缺失,CMake可能会在构建过程中失败并显示错误消息。
Create a class called Rational for performing arithmetic with fractions. Use integer variables to represent the private data of the class – the numerator and the denominator. Provide a constructor that enables an object of this class to be initialized when it’s declared. The constructor should store the fraction in reduced form. For example, the fraction 2/4 would be stored in the object as 1 in the numerator and 2 in the denominator. In order to compute the reduced form, you need to write a reduction function which uses the Euclidean algorithm to get the greatest common divisor (GCD) of the numerator and denominator first and then divides GCD to get the reduced numerator and denominator. Provide public member functions that perform each of the following tasks: (a) Subtract a Rational number from the other Rational number. The result should be stored in reduced form. (b) Divide a Rational number by the other Rational number. The result should be stored in reduced form. (c) Print Rational numbers in the form a/b, where a is the numerator and b is the denominator. (d)Compare two Rational numbers to make sure which one is smaller or they are equal. (1 for the first number, 2 for the second number and 0 if they are equal) Please also write a main function to prompt the user to input two Rational numbers . Subtract one number from the other from these two numbers using (a) and then print the result using (c). Divide one number from the other from these two numbers using (b) and then print the result using (c). Compare these two Rational numbers using (d) and indicate which one is smaller or they are equal. 用c++5.11寫出,且使用using namespace std;
以下是符合要求的C++ 代码:
```
#include <iostream>
using namespace std;
class Rational {
private:
int numerator, denominator;
int gcd(int a, int b) {
if (b == 0) return a;
return gcd(b, a % b);
}
public:
Rational(int num = 0, int den = 1) {
int divisor = gcd(num, den);
numerator = num / divisor;
denominator = den / divisor;
}
Rational operator- (Rational other) {
int num = numerator * other.denominator - other.numerator * denominator;
int den = denominator * other.denominator;
return Rational(num, den);
}
Rational operator/ (Rational other) {
int num = numerator * other.denominator;
int den = denominator * other.numerator;
return Rational(num, den);
}
void print() {
cout << numerator << "/" << denominator;
}
int compare(Rational other) {
int num1 = numerator * other.denominator;
int num2 = other.numerator * denominator;
if (num1 < num2) return 1;
if (num1 > num2) return 2;
return 0;
}
};
int main() {
Rational r1, r2;
cout << "Enter the first rational number (numerator denominator): ";
cin >> r1;
cout << "Enter the second rational number (numerator denominator): ";
cin >> r2;
Rational diff = r1 - r2;
cout << "The difference is ";
diff.print();
cout << endl;
Rational quotient = r1 / r2;
cout << "The quotient is ";
quotient.print();
cout << endl;
int result = r1.compare(r2);
if (result == 1) {
cout << "The first number is smaller." << endl;
} else if (result == 2) {
cout << "The second number is smaller." << endl;
} else {
cout << "The two numbers are equal." << endl;
}
return 0;
}
```
请注意,此代码使用了重载运算符-和/来实现两个Rational数的减法和除法。此外,它还定义了一个比较函数来比较两个Rational数的大小。在主函数中,我们首先提示用户输入两个Rational数,然后执行所需的操作并输出结果。
阅读全文
相关推荐
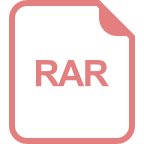
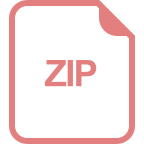
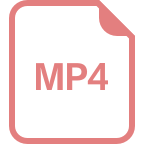
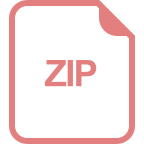
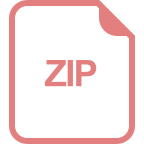
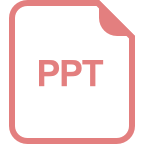
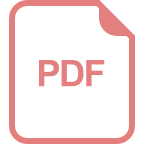
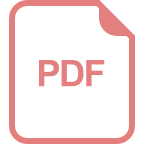
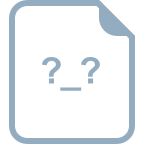
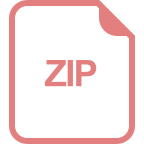
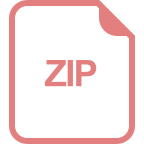
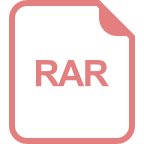



