python 基于傅里叶变换实现低通滤波图像变换
时间: 2023-06-23 14:53:41 浏览: 54
可以使用Python中的numpy和OpenCV库来实现基于傅里叶变换的低通滤波图像变换。下面是一个简单的实现步骤:
1. 读取图像并转换为灰度图像。
```python
import cv2
import numpy as np
# 读取图像并转换为灰度图像
img = cv2.imread('input.jpg')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
```
2. 对灰度图像进行傅里叶变换,并将零频率分量移到频谱中心。
```python
# 对灰度图像进行傅里叶变换
f = np.fft.fft2(gray)
# 将零频率分量移到频谱中心
fshift = np.fft.fftshift(f)
```
3. 构建低通滤波器并将其应用于频谱。
```python
# 构建低通滤波器
rows, cols = gray.shape
crow, ccol = rows // 2, cols // 2
mask = np.zeros((rows, cols), np.uint8)
mask[crow - 30 : crow + 30, ccol - 30 : ccol + 30] = 1
fshift = fshift * mask
# 将零频率分量移回原来位置
f_ishift = np.fft.ifftshift(fshift)
# 对变换后的频谱进行傅里叶逆变换
img_back = np.fft.ifft2(f_ishift)
img_back = np.abs(img_back)
```
4. 显示变换后的图像。
```python
# 显示变换后的图像
cv2.imshow('Input Image', gray)
cv2.imshow('Low Pass Filtered Image', img_back)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
相关推荐
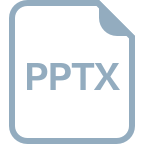
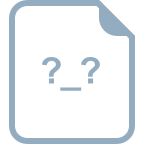
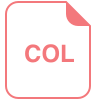
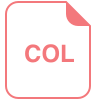
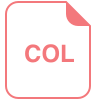
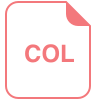
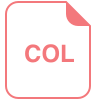









