c++主函数定义数组,自定义函数实现数组的输入、输出、排序、插入
时间: 2023-08-03 14:03:28 浏览: 179
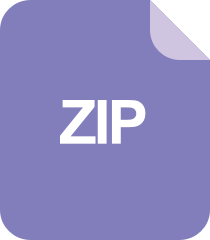
c++实现快速排序源码.zip
下面是一个示例代码,实现了主函数定义数组、自定义函数实现数组的输入、输出、排序、插入:
```c++
#include<iostream>
using namespace std;
void input(int arr[], int n); // 输入函数声明
void output(int arr[], int n); // 输出函数声明
void sort(int arr[], int n); // 排序函数声明
void insert(int arr[], int& n, int pos, int val); // 插入函数声明
int main(){
const int MAXSIZE = 100;
int arr[MAXSIZE];
int n; // 数组元素个数
cout << "请输入数组的元素个数:";
cin >> n;
input(arr, n); // 调用输入函数
output(arr, n); // 调用输出函数
sort(arr, n); // 调用排序函数
output(arr, n); // 再次输出,查看排序结果
int pos, val; // 插入位置和值
cout << "请输入要插入的位置和值(用空格隔开):";
cin >> pos >> val;
insert(arr, n, pos, val); // 调用插入函数
output(arr, n); // 再次输出,查看插入结果
return 0;
}
void input(int arr[], int n){
cout << "请依次输入数组的元素:";
for(int i = 0; i < n; i++){
cin >> arr[i];
}
}
void output(int arr[], int n){
cout << "数组元素为:";
for(int i = 0; i < n; i++){
cout << arr[i] << " ";
}
cout << endl;
}
void sort(int arr[], int n){
for(int i = 0; i < n - 1; i++){
for(int j = 0; j < n - i - 1; j++){
if(arr[j] > arr[j + 1]){
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
void insert(int arr[], int& n, int pos, int val){
if(pos < 0 || pos >= n){ // 判断插入位置是否合法
cout << "插入位置不合法!" << endl;
return;
}
for(int i = n - 1; i >= pos; i--){ // 元素后移
arr[i + 1] = arr[i];
}
arr[pos] = val; // 插入元素
n++; // 数组元素个数加一
}
```
在这个示例代码中:
- 主函数定义了一个长度为100的数组,以及数组元素个数n。
- 调用了自定义输入函数input、输出函数output、排序函数sort、插入函数insert。
- 输入函数input使用for循环依次输入数组的元素。
- 输出函数output使用for循环输出数组的元素。
- 排序函数sort使用冒泡排序算法对数组进行排序。
- 插入函数insert先判断插入位置是否合法,然后使用for循环将插入位置之后的元素全部后移一位,最后将要插入的元素插入到指定位置,并且数组元素个数n加一。
你可以根据自己的需要修改函数实现,例如使用其他排序算法、插入方式等等。
阅读全文
相关推荐
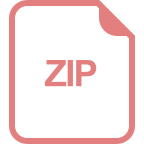
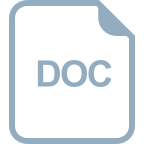















