spring 配置 mybatis-plus
时间: 2023-05-31 15:04:56 浏览: 268
1. 添加 mybatis-plus 依赖
```xml
<dependency>
<groupId>com.baomidou.mybatisplus</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.4.3.2</version>
</dependency>
```
2. 配置数据源
```yml
spring:
datasource:
driver-class-name: com.mysql.cj.jdbc.Driver
url: jdbc:mysql://localhost:3306/mybatisplus?useSSL=false&useUnicode=true&characterEncoding=utf-8&serverTimezone=Asia/Shanghai
username: root
password: root
```
3. 配置 mybatis-plus
```java
@Configuration
@MapperScan("com.example.mapper")
public class MybatisPlusConfig {
@Autowired
private DataSource dataSource;
/**
* 分页插件
*/
@Bean
public PaginationInterceptor paginationInterceptor() {
PaginationInterceptor paginationInterceptor = new PaginationInterceptor();
// 设置方言
paginationInterceptor.setDbType(DbType.MYSQL);
return paginationInterceptor;
}
/**
* mybatis-plus SQL执行效率插件【生产环境可以关闭】
*/
@Bean
@Profile({"dev", "test"}) // 设置 dev test 环境开启
public PerformanceInterceptor performanceInterceptor() {
PerformanceInterceptor performanceInterceptor = new PerformanceInterceptor();
performanceInterceptor.setMaxTime(1000); // SQL执行最大时长,超过自动停止运行,有助于发现问题。
performanceInterceptor.setFormat(true); // SQL是否格式化,默认false。
return performanceInterceptor;
}
@Bean
public MybatisSqlSessionFactoryBean mybatisSqlSessionFactoryBean() {
MybatisSqlSessionFactoryBean sessionFactory = new MybatisSqlSessionFactoryBean();
sessionFactory.setDataSource(dataSource);
// 设置mapper文件扫描路径
sessionFactory.setMapperLocations(new PathMatchingResourcePatternResolver().getResources("classpath*:mapper/*.xml"));
// 设置mybatis配置文件路径
sessionFactory.setConfigLocation(new ClassPathResource("mybatis-config.xml"));
// 设置分页插件和SQL执行效率插件
sessionFactory.setPlugins(new Interceptor[]{paginationInterceptor(), performanceInterceptor()});
return sessionFactory;
}
}
```
4. 配置实体类和 Mapper
```java
@Data
public class User {
private Long id;
private String name;
private Integer age;
private String email;
private Date createTime;
private Date updateTime;
}
@Mapper
public interface UserMapper extends BaseMapper<User> {
}
```
5. 使用示例
```java
@Service
public class UserServiceImpl implements UserService {
@Autowired
private UserMapper userMapper;
@Override
public List<User> list() {
return userMapper.selectList(null);
}
@Override
public User getById(Long id) {
return userMapper.selectById(id);
}
@Override
public boolean save(User user) {
return userMapper.insert(user) > 0;
}
@Override
public boolean update(User user) {
return userMapper.updateById(user) > 0;
}
@Override
public boolean removeById(Long id) {
return userMapper.deleteById(id) > 0;
}
}
```
阅读全文
相关推荐
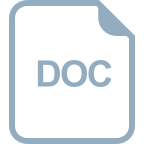
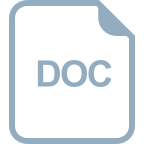
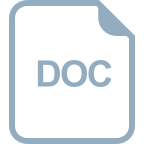
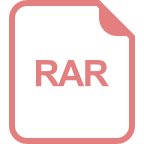
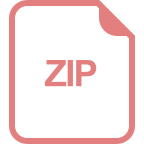
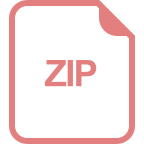
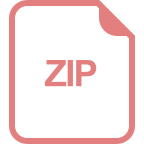



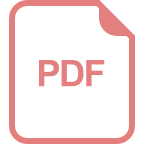
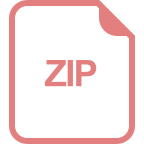
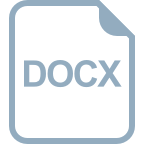
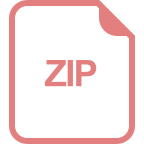
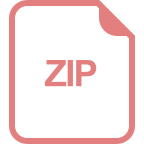



