使用VUE在图片上划区域,如何让线条看起来更舒适美观
时间: 2024-09-14 13:15:04 浏览: 41
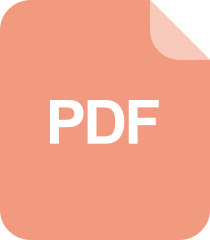
vue实现简易图片左右旋转,上一张,下一张组件案例
在Vue中实现在图片上划区域并让线条看起来更加舒适美观,可以通过以下步骤和技巧来完成:
1. 使用HTML5的`<canvas>`元素:首先,在Vue组件的模板中嵌入一个`<canvas>`元素,并通过Vue的绑定属性确保它的宽高与图片匹配。
2. 监听鼠标事件:在Vue的methods中添加鼠标事件处理函数,如`mousedown`, `mousemove`, 和 `mouseup`,来追踪用户的绘图动作。
3. 使用JavaScript绘图:在`mousemove`事件的回调函数中,使用`canvas`的`getContext('2d')`方法获取绘图上下文,并调用绘图函数来绘制线条。例如,可以使用`lineTo`和`stroke`方法来绘制线条。
4. 美化线条:为了使线条看起来更舒适美观,可以调整以下属性:
- 使用`strokeStyle`来设置线条的颜色。
- 使用`lineWidth`属性来设置线条的宽度。
- 使用`lineCap`和`lineJoin`属性来定义线条的端点和连接点样式。
- 使用`miterLimit`属性来限制斜接处的长度,以避免过尖的转角。
- 使用`shadowBlur`、`shadowColor`等属性为线条添加阴影效果,增强立体感。
5. 清除和重绘:如果需要重绘或清除之前绘制的区域,可以在`mousedown`和`mouseup`事件中添加清除画布的逻辑,并在`mouseup`之后重新绘制当前的线条。
6. Vue响应式更新:确保在用户绘制或修改线条时,Vue的响应式系统能够捕捉到变化并更新DOM。
下面是实现该功能的简单示例代码:
```html
<template>
<div>
<canvas ref="drawingCanvas" @mousedown="startDrawing" @mousemove="draw" @mouseup="stopDrawing"></canvas>
</div>
</template>
<script>
export default {
data() {
return {
isDrawing: false,
startX: 0,
startY: 0,
lastX: 0,
lastY: 0,
ctx: null,
};
},
mounted() {
this.ctx = this.$refs.drawingCanvas.getContext('2d');
this.$refs.drawingCanvas.width = this.$refs.drawingCanvas.offsetWidth;
this.$refs.drawingCanvas.height = this.$refs.drawingCanvas.offsetHeight;
},
methods: {
startDrawing(e) {
this.isDrawing = true;
this.startX = e.offsetX;
this.startY = e.offsetY;
this.lastX = e.offsetX;
this.lastY = e.offsetY;
// 可以在这里做一些初始化的操作,比如设置画笔颜色、宽度等
},
draw(e) {
if (!this.isDrawing) return;
const currentX = e.offsetX;
const currentY = e.offsetY;
this.ctx.beginPath();
this.ctx.strokeStyle = 'rgba(0, 0, 0, 1)';
this.ctx.lineWidth = 2;
this.ctx.lineCap = 'round';
this.ctx.lineJoin = 'round';
this.ctx.moveTo(this.startX, this.startY);
this.ctx.lineTo(currentX, currentY);
this.ctx.stroke();
this.lastX = currentX;
this.lastY = currentY;
},
stopDrawing() {
this.isDrawing = false;
}
}
};
</script>
```
阅读全文
相关推荐
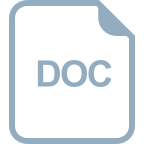
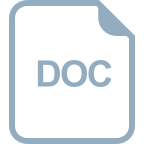
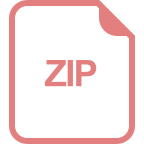
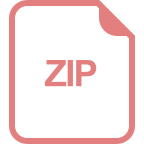
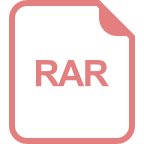
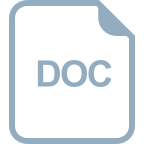
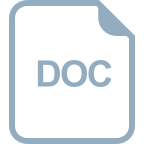
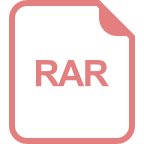
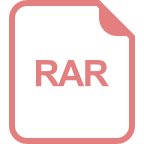
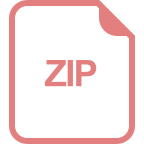
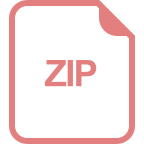
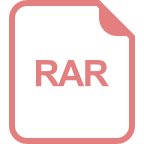