C语言实现ipv4转化
时间: 2024-12-17 21:31:04 浏览: 7
在C语言中,IPv4地址通常是一个32位的无符号整数,可以使用结构体和指针来表示和操作。以下是一个简单的示例,展示如何使用C语言实现IPv4地址的存储和基本的操作:
```c
#include <stdio.h>
#include <netinet/in.h> // 包含IP头文件
// 定义一个IPv4地址结构
struct IPv4Address {
uint32_t address; // 32位无符号整数代表IP地址
};
// 将十进制形式的IP转换为二进制
void decimalToBinary(struct IPv4Address *ip, uint32_t decimal) {
char binary[4][4];
for (int i = 0; i < 4; ++i) {
binary[i] = (char)(decimal % 256);
decimal /= 256;
}
ip->address = inet_addr("0."s + binary[0] + "."s + binary[1] + "."s + binary[2] + "."s + binary[3]);
}
// 显示IPv4地址
void printIPv4(struct IPv4Address ip) {
printf("IPv4 Address: %d.%d.%d.%d\n", ip.address & 0xFF, (ip.address >> 8) & 0xFF, (ip.address >> 16) & 0xFF, ip.address >> 24);
}
int main() {
struct IPv4Address ip;
uint32_t decimal_ip = 192 << 24 | 168 << 16 | 0 << 8 | 1;
// 转换并显示IPv4地址
decimalToBinary(&ip, decimal_ip);
printIPv4(ip);
return 0;
}
```
在这个例子中,`decimalToBinary`函数将给定的十进制IP地址转换为二进制形式,然后赋值给`IPv4Address`结构的`address`字段。`printIPv4`函数则用于打印出这个IPv4地址。
注意:在实际应用中,更常见的是直接使用`inet_addr`和`inet_ntoa`函数处理IPv4地址字符串,而不是手动转换。上面的例子仅为了教学目的演示基础原理。
阅读全文
相关推荐
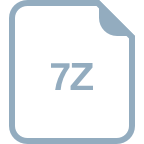
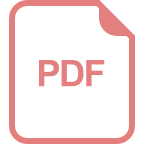
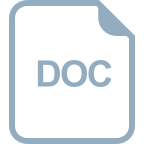
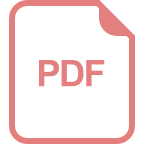
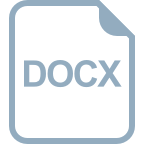
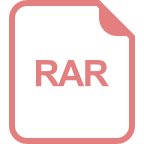
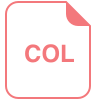
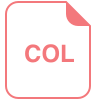
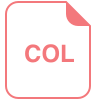
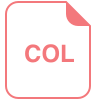




