string texttoinser 方式插入新文本
时间: 2024-10-17 17:14:00 浏览: 2
在C#中,如果你有一个字符串变量`textToInsert`,并想将其插入到已存在的`TextBox`或其他可编辑控件中的指定位置,你可以使用字符串拼接或者`Substring`+`Insert`方法来实现。假设`currentText`是现有文本,`insertionPoint`是插入位置,可以这样做:
```csharp
string currentText = "这是现有的文本";
string textToInsert = "要插入的新文本";
// 使用Substring和Insert方法
int newPosition = insertionPoint + textToInsert.Length; // 新位置计算
string newText = currentText.Substring(0, insertionPoint) + textToInsert + currentText.Substring(insertionPoint);
// 或者直接使用字符串连接
// string newText = currentText.Substring(0, insertionPoint) + textToInsert + currentText.Substring(insertionPoint);
// 将新文本应用到TextBox上
yourTextBox.Text = newText;
```
这里的关键在于先切分原文本成两部分,并将插入的新文本插入到合适的地方,然后再将这两部分拼接起来。
阅读全文
相关推荐
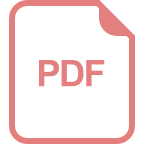
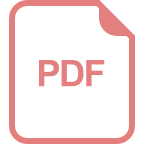
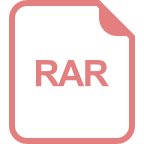
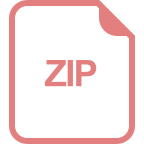
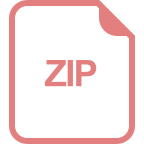
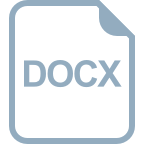
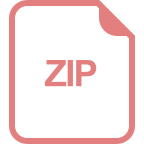
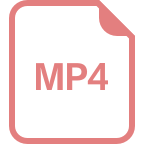
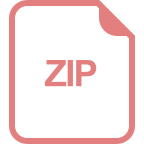