写个C#脚本工具,对比两个ipa包的info.plsit文件的相识度,怎么对比
时间: 2024-10-27 16:08:04 浏览: 9
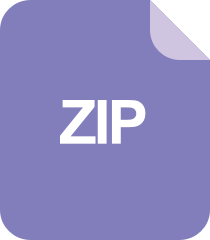
C#全盘查找图片对比相识度 代码 源码
在C#中编写一个脚本来比较两个IPA包(iOS应用的安装包)的`Info.plist`文件相似度,你可以按照以下步骤设计:
1. 首先,你需要通过第三方库解析plist文件,例如`System.IO.Plist`(如果你的目标是.NET Core)或者`TinyIpa`(适用于.NET Framework或旧版本.NET Core,它提供了更方便的解析功能)。
2. 定义一个函数来读取并加载两个`Info.plist`文件的内容。这个函数会返回一个字典或数据结构,其中包含每个键值对。
```csharp
using TinyIpa;
using System.Collections.Generic;
public Dictionary<string, string> ComparePlists(string filePath1, string filePath2)
{
var dict1 = IpaHelper.ReadInfoPlist(filePath1);
var dict2 = IpaHelper.ReadInfoPlist(filePath2);
return new Dictionary<string, string>
{
{ "FilePath1", filePath1 },
{ "FilePath2", filePath2 },
// 这里可以添加更多key-value对,比如逐个比较两个字典是否相等
};
}
```
3. 然后,你需要实现一个算法来计算两个字典的相似度。这可能涉及创建一个哈希集合来存储唯一键值对,然后比较它们的交集。简单来说,可以计算交集大小占其中一个集合大小的比例作为相似度分数。
```csharp
double CalculateSimilarity(Dictionary<string, string> dict1, Dictionary<string, string> dict2)
{
HashSet<(string key, string value)> set1 = new HashSet<(string, string)>(dict1.Select(p => (p.Key, p.Value)));
HashSet<(string key, string value)> set2 = new HashSet<(string, string)>(dict2.Select(p => (p.Key, p.Value)));
int intersectionSize = set1.Intersect(set2).Count;
double similarityScore = (intersectionSize / Math.Max(dict1.Count, dict2.Count)) * 100;
return similarityScore;
}
Dictionary<string, string> result = ComparePlists("path/to/file1.ipa", "path/to/file2.ipa");
double score = CalculateSimilarity(result["FilePath1"], result["FilePath2"]);
```
4. 最后,将结果和得分打印出来,或者根据需要返回给用户。
```csharp
Console.WriteLine($"The two IPA files have a similarity of {score}%.");
```
阅读全文
相关推荐
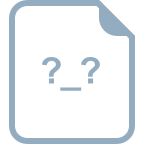
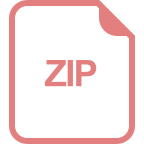















