在C++数据结构中,int a[5]={40,50,60,70,80}用单链表类实现的存储与基本操作,基本操作为:(1)建立含有若干个元素的单链表;(2)对已建立的单链表实现插入、删除、查找(按值、按位置)等操作
时间: 2024-09-23 07:16:20 浏览: 44
在C++中,为了将数组`a[5]`的内容转换为单链表并实现基本操作,我们需要首先创建一个链表节点类`ListNode`,包含一个整数值`value`和一个指向下一个节点的指针`next`。然后,我们构建链表结构并实现插入、删除和查找功能。这里给出一个简单的实现:
```cpp
#include <iostream>
// 定义链表节点
class ListNode {
public:
int value;
ListNode* next;
ListNode(int val) : value(val), next(nullptr) {}
};
// 插入节点到链表末尾
ListNode* insertAtEnd(ListNode* head, int value) {
if (head == nullptr) {
return new ListNode(value);
}
ListNode* current = head;
while (current->next != nullptr) {
current = current->next;
}
current->next = new ListNode(value);
return head;
}
// 删除节点(按值)
ListNode* deleteNode(ListNode* head, int value) {
if (head == nullptr || head->value == value) {
ListNode* temp = head;
head = head->next;
delete temp;
return head;
}
ListNode* current = head;
while (current->next != nullptr && current->next->value != value) {
current = current->next;
}
if (current->next != nullptr) {
ListNode* toDelete = current->next;
current->next = current->next->next;
delete toDelete;
}
return head;
}
// 查找节点(按值)
ListNode* searchNode(ListNode* head, int value) {
ListNode* current = head;
while (current != nullptr) {
if (current->value == value) {
return current;
}
current = current->next;
}
return nullptr; // 如果找不到则返回nullptr
}
// 打印链表
void printList(ListNode* head) {
ListNode* current = head;
while (current != nullptr) {
std::cout << current->value << " -> ";
current = current->next;
}
std::cout << "NULL" << std::endl;
}
int main() {
int arr[] = {40, 50, 60, 70, 80};
ListNode* listHead = nullptr;
for (int i = 0; i < sizeof(arr) / sizeof(arr[0]); ++i) {
listHead = insertAtEnd(listHead, arr[i]);
}
// 打印原始链表
printList(listHead);
// 按值删除节点
listHead = deleteNode(listHead, 60);
printList(listHead);
// 查找节点
ListNode* found = searchNode(listHead, 70);
if (found) {
std::cout << "Found node with value: " << found->value << std::endl;
} else {
std::cout << "Value not found." << std::endl;
}
return 0;
}
```
这个程序首先将数组元素插入到链表的末尾,然后演示了如何删除特定值的节点和查找节点的功能。
阅读全文
相关推荐




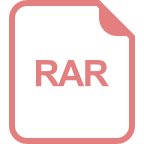


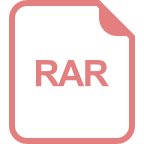

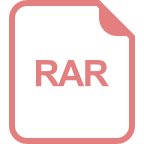








