This simple class contains four instance variables, noting that they are defined as ‘public’. Add more codes as below to instantiate the class Person and initialize some instance variables. class Program { static void Main(string[ ] args) { Person David = new Person(); Person Alice = new Person(); // Initialize David David.age = 21; David.name = "David"; David.weight = 185.4; David.height = 72.3; // Initialize Alice Alice.age = 18; Alice.name = "Alice"; Alice.weight = 125.7; Alice.height = 67.1; // print some values Console.WriteLine("David’s age = {0}; David’s weight = {1}",David.age, David.weight); Console.WriteLine("Alice’s age = {0}; Alice’s weight = {1}", Alice.age, Alice.weight); } } Properties in C#: In the previous, we accessed the characteristics of the Person Class using some public methods such as ‘setXXX(…)’ and ‘getXXX()’. C# gives us a more controlled way to access these data, called properties. Search the internet (‘Baidu’ or ‘Google’) about the property and amend the code above accordingly using the property. (Task 1.3)
时间: 2024-04-16 18:26:03 浏览: 126
class Person
{
public int Age { get; set; }
public string Name { get; set; }
public double Weight { get; set; }
public double Height { get; set; }
}
class Program
{
static void Main(string[] args)
{
Person David = new Person();
Person Alice = new Person();
// Initialize David
David.Age = 21;
David.Name = "David";
David.Weight = 185.4;
David.Height = 72.3;
// Initialize Alice
Alice.Age = 18;
Alice.Name = "Alice";
Alice.Weight = 125.7;
Alice.Height = 67.1;
// Print some values
Console.WriteLine("David’s age = {0}; David’s weight = {1}", David.Age, David.Weight);
Console.WriteLine("Alice’s age = {0}; Alice’s weight = {1}", Alice.Age, Alice.Weight);
}
}
In the modified code, we have replaced the public instance variables with properties in the Person class. Properties provide a more controlled way to access and modify data.
Properties are defined using the `{ get; set; }` syntax. They allow you to get the value of the property using `PropertyName` and set the value using `PropertyName = value`.
In the Main method, we now use the properties `Age`, `Name`, `Weight`, and `Height` to initialize the attributes for David and Alice. When printing the values, we access the property values directly.
Using properties instead of public instance variables provides encapsulation and allows for better control over data access.
阅读全文
相关推荐



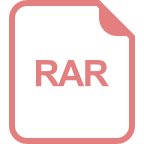


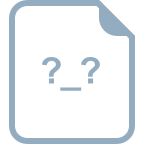






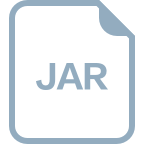