数据结构查找排序实验
时间: 2023-12-27 09:04:31 浏览: 104
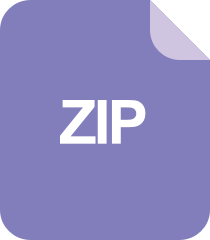
数据结构查找实验
以下是数据结构查找排序实验的步骤和示例:
1. 二叉排序树查找:
- 构造二叉排序树:根据给定的数据,构造一个二叉排序树。
- 查找元素:在二叉排序树中查找指定的元素。
示例:
```python
# 构造二叉排序树
class Node:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
def insert(root, value):
if root is None:
return Node(value)
if value < root.value:
root.left = insert(root.left, value)
else:
root.right = insert(root.right, value)
return root
def construct_tree(data):
root = None
for value in data:
root = insert(root, value)
return root
# 查找元素
def search(root, value):
if root is None or root.value == value:
return root
if value < root.value:
return search(root.left, value)
return search(root.right, value)
# 测试数据
data = [150, 70, 160, 190, 10, 55, 175]
root = construct_tree(data)
# 查找元素
elements = [150, 70, 160, 190, 10, 55, 175]
for element in elements:
result = search(root, element)
if result:
print(f"元素 {element} 在二叉排序树中找到了")
else:
print(f"元素 {element} 在二叉排序树中未找到")
```
2. 二分查找:
- 准备有序数据表:准备一个有序的数据表。
- 二分查找元素:在有序数据表中使用二分查找算法查找指定的元素。
示例:
```python
def binary_search(data, target):
low = 0
high = len(data) - 1
while low <= high:
mid = (low + high) // 2
if data[mid] == target:
return mid
elif data[mid] < target:
low = mid + 1
else:
high = mid - 1
return -1
# 测试数据
data = [10, 55, 70, 150, 160, 175, 190]
elements = [150, 70, 160, 190, 10, 55, 175]
# 二分查找元素
for element in elements:
index = binary_search(data, element)
if index != -1:
print(f"元素 {element} 在数据表中的下标为 {index}")
else:
print(f"元素 {element} 在数据表中未找到")
```
阅读全文
相关推荐
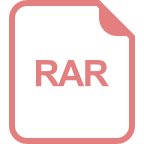
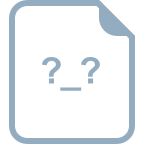
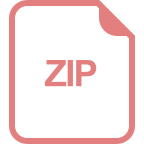
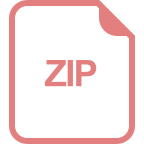
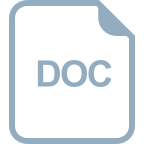
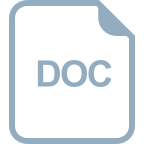
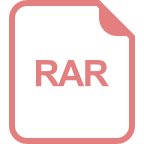
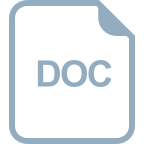
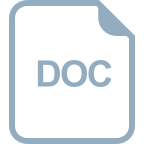
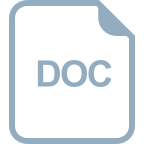
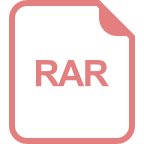
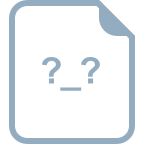
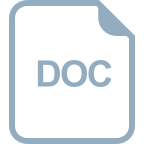
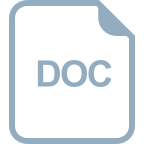
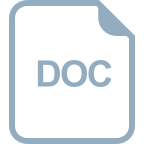
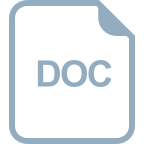