python中re.search的用法
时间: 2023-04-20 08:04:09 浏览: 105
re.search()是Python中正则表达式模块re中的一个函数,用于在字符串中搜索匹配正则表达式的第一个位置,并返回一个匹配对象。如果没有找到匹配项,则返回None。
re.search()的语法如下:
re.search(pattern, string, flags=)
其中,pattern是正则表达式,string是要搜索的字符串,flags是可选参数,用于控制正则表达式的匹配方式。
例如,以下代码使用re.search()函数在字符串中查找匹配正则表达式的第一个位置,并返回匹配对象:
import re
string = "Hello, World!"
pattern = "Hello"
match = re.search(pattern, string)
if match:
print("Match found!")
else:
print("Match not found.")
相关问题
python中re.search.group
re.search.group()是一个re模块中用来匹配字符串的方法,它返回一个MatchObject对象。MatchObject对象包含有关匹配的信息,例如匹配的字符串,匹配的开始和结束位置,以及匹配的组。
re.search.group()方法可以返回匹配的字符串或匹配的组。如果没有指定组号,则返回整个匹配的字符串。如果指定了组号,则返回该组的匹配字符串。
例如,以下代码将查找字符串中的第一个数字,并返回该数字:
```
import re
string = "The quick brown fox jumps over the 123 lazy dog"
match = re.search(r'\d+', string)
if match:
print("Match found!")
print("Matched string: ", match.group())
else:
print("Match not found.")
```
输出:
```
Match found!
Matched string: 123
```
在这个例子中,re.search()方法被用来查找字符串中的第一个数字。如果找到了匹配,就会创建一个MatchObject对象。然后,我们使用match.group()方法来返回匹配的字符串,即数字"123"。
python中re.search函数
re.search函数是Python中re模块提供的一个用于在字符串中搜索匹配模式的函数。它的语法如下:
```python
re.search(pattern, string, flags=0)
```
其中,`pattern`是正则表达式模式,`string`是要进行匹配的字符串,`flags`是可选的匹配模式标志。该函数会在字符串中搜索第一个与模式匹配的子串,并返回一个匹配对象。如果找到匹配,则可以通过匹配对象的方法和属性来获取匹配的信息。
例如,我们可以使用re.search函数来查找字符串中是否包含指定的模式:
```python
import re
string = "Hello, world!"
pattern = "world"
match = re.search(pattern, string)
if match:
print("Pattern found!")
else:
print("Pattern not found.")
```
在上述示例中,re.search函数会搜索字符串`string`中是否包含模式`pattern`,如果找到匹配则返回一个匹配对象。通过判断匹配对象是否存在,我们可以确定是否找到了指定的模式。
阅读全文
相关推荐
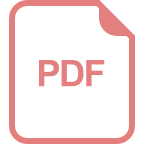
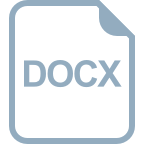
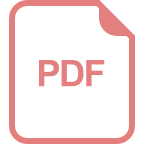




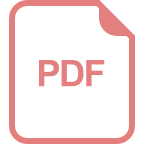




