python如何调用其他函数中的变量
时间: 2024-10-08 14:17:43 浏览: 44
在Python中,当你在一个函数内部定义了一个变量,并希望在另一个函数中访问它,通常有以下几种方式:
1. **全局变量**:如果这个变量是在函数外部定义的全局变量,那么所有函数都可以直接访问。只需确保在调用函数之前已经定义了全局变量。
```python
global_var = "I'm a global variable"
def func1():
print(global_var)
def func2():
print(global_var)
func1()
func2()
```
2. **参数传递**:如果你想要函数间临时共享数据,可以将变量作为参数传递给需要它的函数。
```python
def set_value(value):
my_variable = value
def print_value(func):
return func()
set_value("Hello")
print(print_value(lambda: my_variable)) # 输出 "Hello"
```
3. **返回值**:函数可以在内部计算出结果并返回,接收方可以直接赋值给所需的变量。
```python
def calculate():
result = "Calculated result"
return result
my_variable = calculate()
print(my_variable) # 输出 "Calculated result"
```
4. **非局部作用域**:在一些特定上下文,如`lambda`表达式、列表推导式等,可能会隐含地创建临时作用域,这时变量仅在该范围内可见。若需要长期保留,需明确使用`nonlocal`关键字。
```python
def outer_func():
inner_variable = "From outer function"
def inner_func():
nonlocal inner_variable
inner_variable = "Inner function modified"
inner_func()
print(inner_variable) # 输出 "Inner function modified"
outer_func()
```
阅读全文
相关推荐




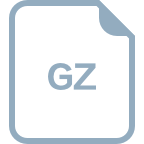











