() => props.editable && props.closable !== false && !props.tab?.disabled, 如何改写成显示关闭按钮
时间: 2024-03-22 07:42:04 浏览: 60
你可以使用这个箭头函数来确定选项卡是否应该显示关闭按钮。如果返回值为 true,则应该显示关闭按钮,否则不应该显示。
下面是一个示例代码:
```
const shouldShowCloseButton = () => {
return props.closable !== false && !props.tab?.disabled;
}
// 在组件中根据 shouldShowCloseButton 函数的返回值来确定是否显示关闭按钮
<button className="close-button" style={{ display: shouldShowCloseButton() ? 'block' : 'none' }} onClick={handleClose}>Close</button>
```
在这个示例中,我们定义了一个名为 `shouldShowCloseButton` 的函数,它返回一个布尔值。这个函数使用了原来的箭头函数中的 `closable` 和 `tab.disabled` 属性,但是没有使用 `editable` 属性,因为它只影响选项卡是否可以编辑,而不影响是否显示关闭按钮。然后,我们在组件中使用 `shouldShowCloseButton` 函数来决定是否显示关闭按钮。
注意,实际显示关闭按钮的方式可能会根据你的具体组件实现而有所不同。在上面的示例中,我们使用了一个带有 `display` 样式的按钮来控制是否显示关闭按钮,但是你的组件实现可能会使用不同的方式来实现相同的效果。
相关问题
() => props.editable && props.closable !== false && !props.tab?.disabled,
这段代码看起来是一个函数,它返回一个布尔值。如果`props.editable`为真且`props.closable`不为`false`且`props.tab.disabled`不存在或为`false`,则返回`true`,否则返回`false`。
在tsx文件中,可以这样使用这个函数:
```tsx
import React from 'react';
interface Props {
editable?: boolean;
closable?: boolean;
tab?: {
disabled?: boolean;
};
}
const shouldRenderTab = (props: Props) => {
return (
props.editable &&
props.closable !== false &&
!props.tab?.disabled
);
};
export default function MyComponent(props: Props) {
const shouldRender = shouldRenderTab(props);
return (
<div>
{shouldRender && <div>Render Tab</div>}
<p>Other content...</p>
</div>
);
}
```
在这个例子中,我们定义了一个名为`Props`的接口来描述组件的props。定义了一个名为`shouldRenderTab`的函数,该函数接受一个`Props`类型的参数并返回一个布尔值。在组件中,我们调用`shouldRenderTab`函数并传入`props`参数,以获取`shouldRender`的值。最后,我们可以根据`shouldRender`的值来决定是否渲染选项卡。
const shouldShowCloseButton = () => { return props.closable !== false && !props.tab?.disabled; } 在tsx里如何读取值
在tsx文件中,你可以这样读取`shouldShowCloseButton`函数的返回值:
```tsx
import React from 'react';
interface Props {
closable?: boolean;
tab?: {
disabled?: boolean;
};
}
const shouldShowCloseButton = (props: Props) => {
return props.closable !== false && !props.tab?.disabled;
};
export default function MyComponent(props: Props) {
const showCloseButton = shouldShowCloseButton(props);
return (
<div>
{showCloseButton && <button>Close</button>}
<p>Other content...</p>
</div>
);
}
```
在该例子中,我们定义了一个名为`Props`的接口来描述组件的props。我们定义了一个名为`shouldShowCloseButton`的函数,该函数接受一个`Props`类型的参数并返回一个布尔值。在组件中,我们调用`shouldShowCloseButton`函数并传入`props`参数,以获取`showCloseButton`的值。最后,我们可以根据`showCloseButton`的值来决定是否渲染关闭按钮。
阅读全文
相关推荐
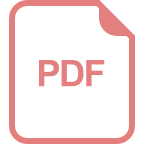

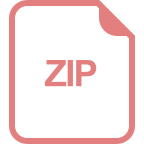
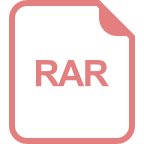
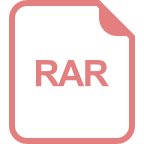
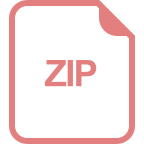
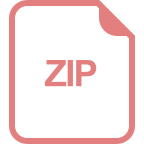
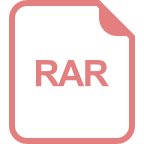
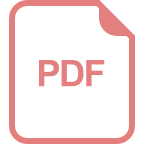
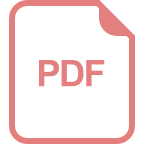
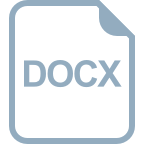
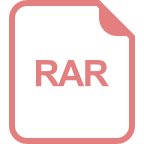
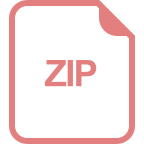
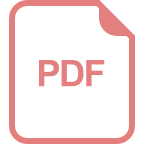