java多线程排序300个随机数的代码
时间: 2023-10-26 13:21:14 浏览: 83
以下是一个简单的Java多线程排序300个随机数的代码示例:
```
import java.util.Arrays;
import java.util.Random;
public class MultiThreadedSort {
public static void main(String[] args) {
int[] nums = new int[300];
Random rand = new Random();
for (int i = 0; i < 300; i++) {
nums[i] = rand.nextInt(1000);
}
SortThread t1 = new SortThread(Arrays.copyOfRange(nums, 0, 100));
SortThread t2 = new SortThread(Arrays.copyOfRange(nums, 100, 200));
SortThread t3 = new SortThread(Arrays.copyOfRange(nums, 200, 300));
t1.start();
t2.start();
t3.start();
try {
t1.join();
t2.join();
t3.join();
} catch (InterruptedException e) {
e.printStackTrace();
}
int[] sortedNums = new int[300];
System.arraycopy(t1.getNums(), 0, sortedNums, 0, 100);
System.arraycopy(t2.getNums(), 0, sortedNums, 100, 100);
System.arraycopy(t3.getNums(), 0, sortedNums, 200, 100);
Arrays.sort(sortedNums);
System.out.println(Arrays.toString(sortedNums));
}
}
class SortThread extends Thread {
private int[] nums;
public SortThread(int[] nums) {
this.nums = nums;
}
public int[] getNums() {
return nums;
}
@Override
public void run() {
Arrays.sort(nums);
}
}
```
阅读全文
相关推荐
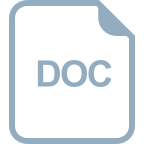
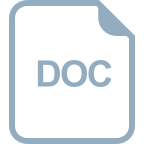
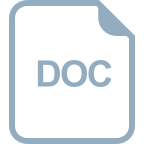
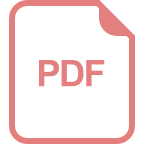
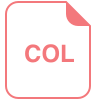
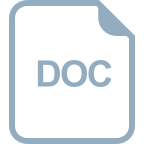
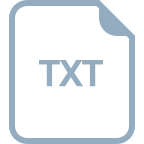
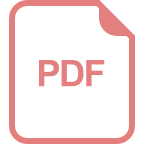
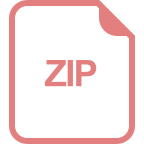
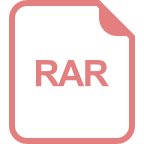
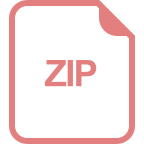
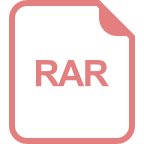
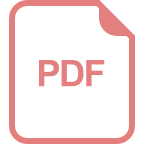
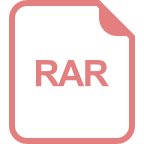
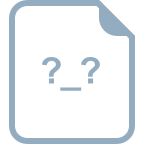
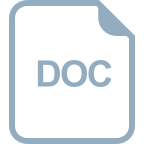
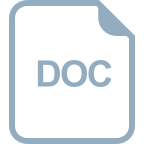
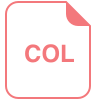
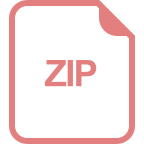
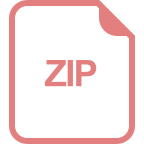