数据结构用devc++怎么敲一个完整代码
时间: 2024-09-09 17:03:52 浏览: 57
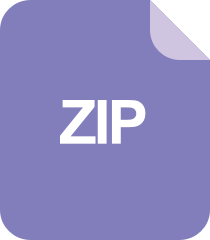
用DEV C++做的小游戏源代码(1)
使用 Dev-C++ 编写一个完整的数据结构代码,首先需要确定你要实现哪种数据结构,比如链表、栈、队列等。这里我以最简单的单链表为例,给出一个在 Dev-C++ 中创建和操作单链表的基本代码框架。
```cpp
#include <iostream>
// 定义链表节点结构体
struct ListNode {
int data; // 数据域
ListNode* next; // 指针域,指向下一个节点
};
// 创建新节点
ListNode* createNode(int value) {
ListNode* newNode = new ListNode();
if (newNode) {
newNode->data = value;
newNode->next = NULL;
}
return newNode;
}
// 向链表末尾添加节点
void appendNode(ListNode*& head, int value) {
ListNode* newNode = createNode(value);
if (!head) {
head = newNode;
return;
}
ListNode* current = head;
while (current->next) {
current = current->next;
}
current->next = newNode;
}
// 打印链表
void printList(ListNode* head) {
ListNode* current = head;
while (current) {
std::cout << current->data << " ";
current = current->next;
}
std::cout << std::endl;
}
// 主函数
int main() {
ListNode* head = NULL; // 初始化链表头指针为NULL
// 向链表添加数据
appendNode(head, 1);
appendNode(head, 2);
appendNode(head, 3);
// 打印链表
printList(head);
// 清理链表内存(释放节点)
ListNode* current = head;
while (current) {
ListNode* next = current->next;
delete current;
current = next;
}
return 0;
}
```
以上代码实现了一个简单的单链表添加和打印功能。接下来,我提出几个相关问题,帮助你更好地理解和使用数据结构:
阅读全文
相关推荐
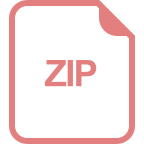
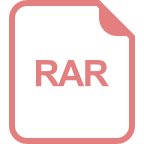
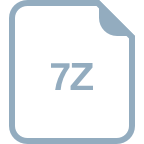
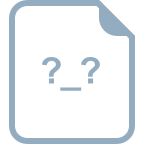
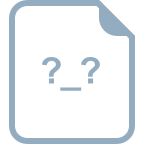
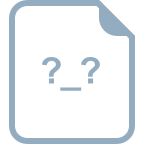
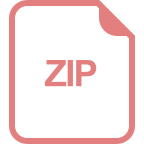
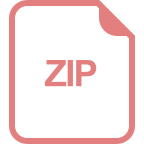
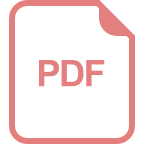
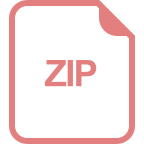
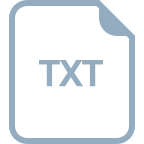