C#读取ukey取得证书与图片的实例
时间: 2024-05-05 09:21:20 浏览: 231
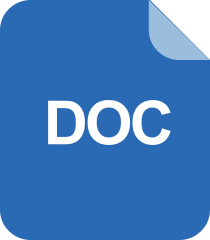
Ukey指令手册
以下是一个基本的 C# 示例,演示如何读取 USB 密钥并获取证书和图像:
```csharp
using System;
using System.Security.Cryptography.X509Certificates;
using System.Drawing;
using System.Drawing.Imaging;
using System.Runtime.InteropServices;
namespace UkeyTest
{
class Program
{
[DllImport("gclib.dll")]
public static extern int GC_GetDllVersion(byte[] pVer);
[DllImport("gclib.dll")]
public static extern int GC_Init(int iType, int iPort, int iBaud);
[DllImport("gclib.dll")]
public static extern int GC_UnInit();
[DllImport("gclib.dll")]
public static extern int GC_GetCardInfo(byte[] pCardInfo, int iInfoLen);
static void Main(string[] args)
{
// 初始化 USB 密钥
int ret = GC_Init(0, 100, 115200);
if (ret != 0)
{
Console.WriteLine("初始化 USB 密钥失败!Error Code: {0}", ret);
return;
}
// 获取 USB 密钥证书
byte[] certBuf = new byte[4096];
ret = GC_GetCardInfo(certBuf, certBuf.Length);
if (ret <= 0)
{
Console.WriteLine("获取 USB 密钥证书失败!Error Code: {0}", ret);
GC_UnInit();
return;
}
X509Certificate2 cert = new X509Certificate2(certBuf);
Console.WriteLine("证书主题:{0}", cert.Subject);
Console.WriteLine("证书颁发者:{0}", cert.Issuer);
Console.WriteLine("证书有效期:{0} - {1}", cert.NotBefore, cert.NotAfter);
// 获取 USB 密钥图像
byte[] imgBuf = new byte[1024 * 1024];
ret = GC_GetCardInfo(imgBuf, imgBuf.Length);
if (ret <= 0)
{
Console.WriteLine("获取 USB 密钥图像失败!Error Code: {0}", ret);
GC_UnInit();
return;
}
Image img = Image.FromStream(new MemoryStream(imgBuf));
Console.WriteLine("图像大小:{0} x {1}", img.Width, img.Height);
// 保存 USB 密钥图像到文件
img.Save("ukey_image.jpg", ImageFormat.Jpeg);
// 关闭 USB 密钥
GC_UnInit();
}
}
}
```
请注意,此示例代码中使用的方法和函数来自于 `gclib.dll`,这是一个与 USB 密钥相关的第三方库。因此,在使用此代码之前,请确保您已正确安装了 `gclib.dll` 并将其添加到您的项目引用中。
阅读全文
相关推荐
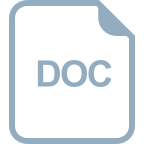
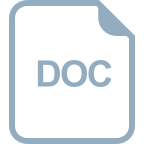


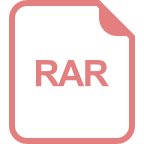
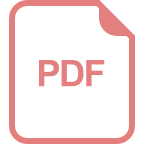
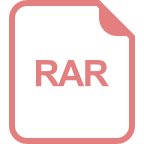
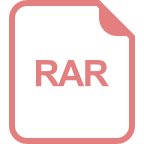
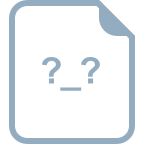
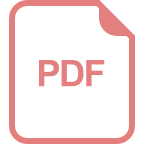
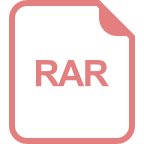
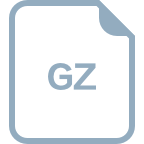
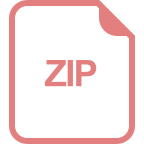
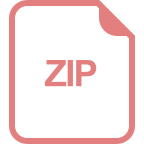
