用python设计小游戏源代码_用Python设计一个经典小游戏、
时间: 2024-04-28 16:20:59 浏览: 9
好的,我可以给你一个经典的Python小游戏——扫雷游戏的源代码。
```python
import random
# 定义方块状态
UNEXPOSED = "-"
EXPOSED = "0"
MINE = "X"
# 定义扫雷游戏类
class MinesweeperGame:
def __init__(self, height, width, mines):
self.height = height
self.width = width
self.mines = mines
self.board = self.create_board(UNEXPOSED)
self.assign_mines(mines)
def create_board(self, value):
return [[value] * self.width for _ in range(self.height)]
def assign_mines(self, mines):
assigned = 0
while assigned < mines:
row = random.randint(0, self.height - 1)
col = random.randint(0, self.width - 1)
if self.board[row][col] != MINE:
self.board[row][col] = MINE
assigned += 1
def get_adjacent_mines(self, row, col):
count = 0
for r in range(max(0, row - 1), min(self.height - 1, row + 1) + 1):
for c in range(max(0, col - 1), min(self.width - 1, col + 1) + 1):
if self.board[r][c] == MINE:
count += 1
return count
def get_all_cells(self):
return [(r, c) for r in range(self.height) for c in range(self.width)]
def get_random_unexposed_cell(self):
unexposed_cells = [(r, c) for r, c in self.get_all_cells() if self.board[r][c] == UNEXPOSED]
return random.choice(unexposed_cells) if unexposed_cells else None
def is_game_over(self):
return any(self.board[r][c] == MINE for r, c in self.get_all_cells() if self.board[r][c] != EXPOSED)
def make_move(self, row, col):
if self.board[row][col] == MINE:
self.board[row][col] = "!" + MINE
else:
self.reveal_cells(row, col)
def reveal_cells(self, row, col):
queue = [(row, col)]
while queue:
r, c = queue.pop(0)
if self.board[r][c] == UNEXPOSED:
self.board[r][c] = str(self.get_adjacent_mines(r, c))
if self.board[r][c] == EXPOSED:
for nr in range(max(0, r - 1), min(self.height - 1, r + 1) + 1):
for nc in range(max(0, c - 1), min(self.width - 1, c + 1) + 1):
if nr != r or nc != c:
queue.append((nr, nc))
def __str__(self):
return "\n".join([" ".join(row) for row in self.board])
# 游戏主函数
def play_game(height=8, width=8, mines=8):
game = MinesweeperGame(height, width, mines)
while not game.is_game_over():
print(game)
row, col = map(int, input("Enter row, col: ").split())
game.make_move(row, col)
print(game)
print("Game over")
# 开始游戏
play_game()
```
这个代码实现了一个简单的扫雷游戏,你可以自定义高度、宽度和地雷数来挑战自己的游戏能力。
相关推荐
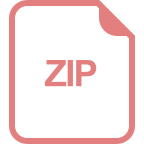
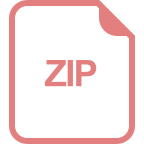
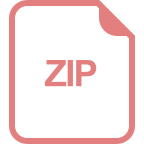










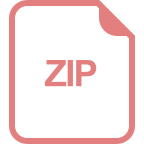