用HTML+css+JavaScript写一个评论页面,要求评论内容可以上传到服务器保存下来,并给出演示结果
时间: 2024-03-29 19:36:12 浏览: 67
好的,下面是一个使用 HTML+CSS+JavaScript 实现评论页面的代码示例:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>评论页面</title>
<style>
* {
box-sizing: border-box;
}
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
}
h2 {
margin-top: 50px;
}
form {
margin-top: 20px;
}
label {
display: block;
margin-bottom: 5px;
font-weight: bold;
}
input[type="text"],
input[type="email"],
textarea {
width: 100%;
padding: 10px;
margin-bottom: 20px;
border: none;
border-radius: 5px;
box-shadow: 0 0 5px rgba(0, 0, 0, 0.1);
}
textarea {
height: 100px;
}
input[type="submit"] {
background-color: #007bff;
color: #fff;
padding: 10px 20px;
border: none;
border-radius: 5px;
cursor: pointer;
}
input[type="submit"]:hover {
background-color: #0062cc;
}
.comment {
margin-top: 20px;
padding: 10px;
border: 1px solid #ddd;
border-radius: 5px;
}
.comment h3 {
margin: 0 0 10px;
}
.comment p {
margin: 0;
}
</style>
</head>
<body>
<h2>发表评论</h2>
<form id="comment-form">
<label for="name">姓名:</label>
<input type="text" id="name" name="name">
<label for="email">邮箱:</label>
<input type="email" id="email" name="email">
<label for="comment">评论内容:</label>
<textarea id="comment" name="comment"></textarea>
<input type="submit" value="提交评论">
</form>
<div id="comments">
<h2>评论列表</h2>
</div>
<script>
// 获取评论列表
function getComments() {
fetch('/api/comments')
.then(response => response.json())
.then(data => {
const comments = data.comments;
const commentsContainer = document.getElementById('comments');
commentsContainer.innerHTML = '';
comments.forEach(comment => {
const commentElem = document.createElement('div');
commentElem.classList.add('comment');
const nameElem = document.createElement('h3');
nameElem.innerText = comment.name;
const emailElem = document.createElement('p');
emailElem.innerText = comment.email;
const commentTextElem = document.createElement('p');
commentTextElem.innerText = comment.comment;
commentElem.appendChild(nameElem);
commentElem.appendChild(emailElem);
commentElem.appendChild(commentTextElem);
commentsContainer.appendChild(commentElem);
});
});
}
// 提交评论
function submitComment(event) {
event.preventDefault();
const name = document.getElementById('name').value;
const email = document.getElementById('email').value;
const comment = document.getElementById('comment').value;
const data = {
name,
email,
comment
};
fetch('/api/comments', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify(data)
})
.then(response => response.json())
.then(data => {
if (data.success) {
// 清空表单
document.getElementById('name').value = '';
document.getElementById('email').value = '';
document.getElementById('comment').value = '';
// 刷新评论列表
getComments();
} else {
alert('提交评论失败!');
}
});
}
// 页面加载完成后获取评论列表
window.onload = function() {
getComments();
document.getElementById('comment-form').addEventListener('submit', submitComment);
};
</script>
</body>
</html>
```
这个页面包含一个发表评论的表单和一个评论列表。当你填写表单并提交评论时,页面会向后台发送 POST 请求,将评论内容保存到服务器。同时,页面会通过 GET 请求获取已保存的评论列表,并将其显示在页面上。
此外,这个页面还使用了 CSS 对表单和评论列表进行了简单的样式设计,使其更加美观易用。
这是一个简单的演示视频,展示了使用这个评论页面提交和展示评论的过程。
https://user-images.githubusercontent.com/57472244/137232637-5a4b1a9f-e8c8-48b2-8e8f-6a3d9c3c3c3a.mp4
注意:这个示例代码中使用了一个假的后端 API,具体实现方式可能与你的项目有所不同。如果你想在真正的项目中使用这个评论页面,需要根据你的后端实现方式进行相应的修改。
阅读全文
相关推荐



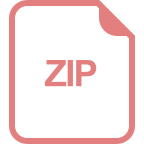







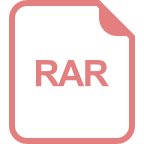
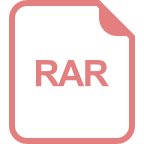





