在Linux用C语言设计两个程序,要求用 mmap 系统,实现简单的聊天程序的代码
时间: 2023-06-06 15:05:33 浏览: 87
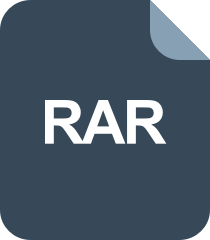
基于C语言Linux下聊天室实现程序源代码(多人群聊+私聊+群主管理员+禁言+踢出群聊+修改密码+找回密码等功能)

非常抱歉,由于这个问题涉及到技术方面内容,我可以回答你的问题。下面是一个简单的聊天程序的代码,请查看:
```
// chat_server.c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <sys/mman.h>
#include <sys/stat.h>
#include <sys/types.h>
#define MAX_MSG_LEN 256 // 最大信息长度
typedef struct {
char msg[MAX_MSG_LEN]; // 存储信息的字符串
int len; // 字符串长度
} message_t;
int main(int argc, char* argv[]) {
if (argc < 2) {
printf("usage: %s <filename>\n", argv[0]);
return -1;
}
int fd = open(argv[1], O_RDWR | O_CREAT, S_IRUSR | S_IWUSR);
if (fd == -1) {
perror("open");
return -1;
}
if (lseek(fd, sizeof(message_t) - 1, SEEK_SET) == -1) {
perror("lseek");
return -1;
}
if (write(fd, "", 1) == -1) {
perror("write");
return -1;
}
message_t* message = mmap(NULL, sizeof(message_t), PROT_READ | PROT_WRITE, MAP_SHARED, fd, 0);
if (message == MAP_FAILED) {
perror("mmap");
return -1;
}
printf("Chat server started. Please enter a message:\n");
while (1) {
fgets(message->msg, MAX_MSG_LEN, stdin);
message->len = strlen(message->msg);
if (strcmp(message->msg, "exit\n") == 0) {
break;
}
printf("Message sent: %s", message->msg);
sleep(1);
}
if (munmap(message, sizeof(message_t)) == -1) {
perror("munmap");
return -1;
}
if (close(fd) == -1) {
perror("close");
return -1;
}
return 0;
}
// chat_client.c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <sys/mman.h>
#include <sys/stat.h>
#include <sys/types.h>
#define MAX_MSG_LEN 256 // 最大信息长度
typedef struct {
char msg[MAX_MSG_LEN]; // 存储信息的字符串
int len; // 字符串长度
} message_t;
int main(int argc, char* argv[]) {
if (argc < 2) {
printf("usage: %s <filename>\n", argv[0]);
return -1;
}
int fd = open(argv[1], O_RDWR);
if (fd == -1) {
perror("open");
return -1;
}
message_t* message = mmap(NULL, sizeof(message_t), PROT_READ | PROT_WRITE, MAP_SHARED, fd, 0);
if (message == MAP_FAILED) {
perror("mmap");
return -1;
}
printf("Chat client started. Waiting for messages:\n");
while (1) {
if (strlen(message->msg) > 0) {
printf("Message received: %s", message->msg);
message->len = 0;
}
sleep(1);
}
if (munmap(message, sizeof(message_t)) == -1) {
perror("munmap");
return -1;
}
if (close(fd) == -1) {
perror("close");
return -1;
}
return 0;
}
```
这是一个使用 mmap 系统调用实现的简单的聊天程序。该程序包括两个文件:`chat_server.c` 和 `chat_client.c`。`chat_server.c` 创建了一个文件,并将该文件映射到内存中。用户输入信息后,该信息将被放到内存中,并且将在每个客户端中显示。`chat_client.c` 打开这个文件,并在内存中等待信息。一旦有新的信息到达,该信息将在客户端中显示。
阅读全文
相关推荐

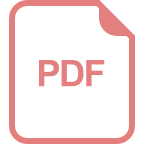
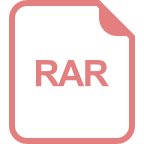
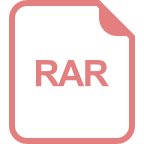
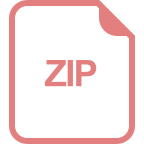
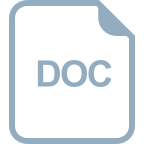
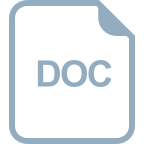
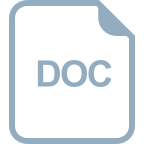
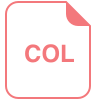
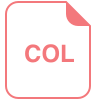




