Operation now in progress
时间: 2024-05-27 17:10:22 浏览: 60
This message usually indicates that a system call has been made, but the operation has not yet completed and is in progress. It is a common error message in programming and can occur in various situations, such as network communication, file input/output, or process management. If you are encountering this error, you may need to wait for the operation to complete or check if there are any issues with your code or system configuration.
相关问题
connet返回Operation now in progress
当`connect`函数返回"Operation now in progress"错误时,表示连接操作已经在进行中。这种情况通常发生在非阻塞套接字上,在连接建立过程中,`connect`函数立即返回并且返回值为-1,同时设置`errno`为EINPROGRESS。
此时,可以使用`select`或`poll`等I/O多路复用函数来检测连接是否已经建立成功。这些函数可以监视套接字上的写操作,当套接字变为可写状态时,表示连接已经建立成功。
以下是一个示例代码,展示如何处理"Operation now in progress"错误:
```c
#include <stdio.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <unistd.h>
#include <fcntl.h>
#include <errno.h>
int main() {
// 创建套接字
int sockfd = socket(AF_INET, SOCK_STREAM, 0);
// 设置目标主机的IP地址和端口号
struct sockaddr_in server_addr;
server_addr.sin_family = AF_INET;
server_addr.sin_port = htons(8080);
server_addr.sin_addr.s_addr = inet_addr("127.0.0.1");
// 将套接字设置为非阻塞模式
int flags = fcntl(sockfd, F_GETFL, 0);
fcntl(sockfd, F_SETFL, flags | O_NONBLOCK);
// 连接到目标主机
int connect_result = connect(sockfd, (struct sockaddr*)&server_addr, sizeof(server_addr));
// 判断连接状态
if (connect_result == -1) {
if (errno == EINPROGRESS) {
// 连接正在进行中,需要使用I/O多路复用函数来检测连接状态
fd_set write_fds;
FD_ZERO(&write_fds);
FD_SET(sockfd, &write_fds);
struct timeval timeout;
timeout.tv_sec = 5; // 设置超时时间为5秒
timeout.tv_usec = 0;
// 等待套接字变为可写状态
int select_result = select(sockfd + 1, NULL, &write_fds, NULL, &timeout);
if (select_result == -1) {
perror("select");
close(sockfd);
return 1;
} else if (select_result == 0) {
fprintf(stderr, "连接超时\n");
close(sockfd);
return 1;
}
// 检查套接字是否可写
if (FD_ISSET(sockfd, &write_fds)) {
// 连接已经建立成功
printf("连接成功\n");
// 进行数据发送和接收操作
// ...
} else {
fprintf(stderr, "连接失败\n");
close(sockfd);
return 1;
}
} else {
perror("连接失败");
// 进行错误处理
close(sockfd);
return 1;
}
}
// 关闭套接字
close(sockfd);
return 0;
}
```
在这个示例中,当`connect`函数返回"Operation now in progress"错误时,会使用`select`函数来等待套接字变为可写状态。如果套接字可写,表示连接建立成功;否则,表示连接失败。
需要注意的是,这只是一种处理方式,具体的实现方法可能因环境和需求而异。建议查阅相关文档和参考资料,以便了解更多关于非阻塞套接字和I/O多路复用的知识。
connect 返回Operation now in progress
如果connect函数返回Operation now in progress错误码(错误码为EINPROGRESS),则表示连接正在进行中,需要等待连接完成后再进行其他操作。这种情况通常发生在非阻塞套接字上,因为在非阻塞模式下,connect函数会立即返回而不是一直阻塞等待连接完成。此时可以使用select、poll、epoll等函数来等待套接字变为可写状态,表示连接已经完成。也可以将套接字设置为阻塞模式,这样connect函数将一直阻塞等待连接完成,直到超时或连接成功。
阅读全文
相关推荐
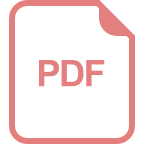
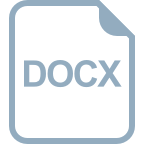
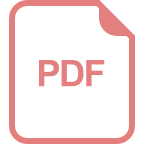













