nodejs连接mongodb数据库,校验用户是否注册过
时间: 2023-06-25 11:02:18 浏览: 116
要连接 MongoDB 数据库,我们需要使用 Node.js 的 mongodb 驱动程序。您可以使用以下步骤在 Node.js 中连接 MongoDB 数据库:
1. 安装 mongodb 驱动程序
```sh
npm install mongodb
```
2. 在 Node.js 中编写连接代码
```js
const MongoClient = require('mongodb').MongoClient;
// Connection URL
const url = 'mongodb://localhost:27017';
// Database Name
const dbName = 'myproject';
// Create a new MongoClient
const client = new MongoClient(url);
// Use connect method to connect to the Server
client.connect(function(err) {
console.log("Connected successfully to server");
const db = client.db(dbName);
// perform actions on the database collection
client.close();
});
```
3. 然后,您可以使用 db.collection() 方法获取集合并执行操作。例如,如果您有一个名为 "users" 的集合,并且要检查用户是否存在,则可以使用以下代码:
```js
// check if user exists
const user = await db.collection('users').findOne({ username: 'myusername' });
if (user) {
console.log('User already exists');
} else {
console.log('User does not exist');
}
```
请注意,上述代码是使用 async/await 语法编写的。如果您不熟悉这些概念,请查看更多有关 Node.js 和 MongoDB 的文档。
阅读全文
相关推荐
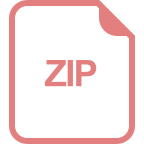
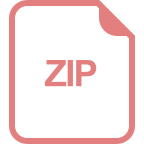
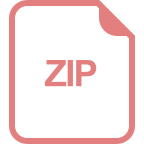
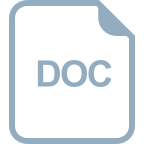
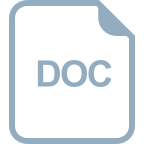
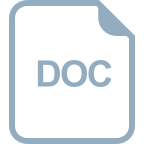
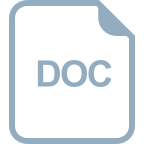
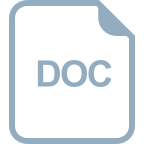
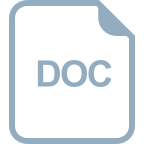
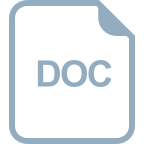
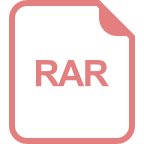
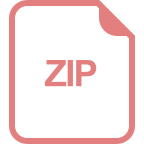
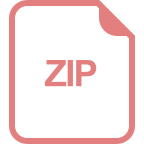
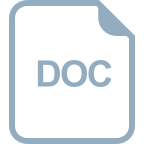
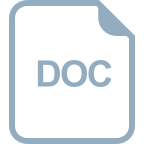
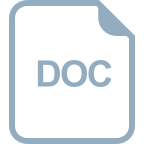
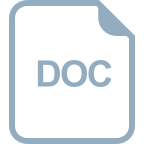
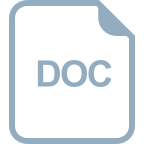
