编写一段java代码获取ppt内部动画
时间: 2024-02-16 08:03:21 浏览: 311
获取PPT内部动画可以使用Apache POI库来解析PPT文件,具体步骤如下:
1. 加载PPT文件,创建XMLSlideShow对象
```
FileInputStream fis = new FileInputStream("test.pptx");
XMLSlideShow ppt = new XMLSlideShow(fis);
```
2. 遍历PPT中的所有幻灯片,获取每页Slide对象
```
for (XSLFSlide slide : ppt.getSlides()) {
// 处理每一页幻灯片
}
```
3. 遍历每个Slide对象中的所有动画,获取每个XSLFShape对象
```
for (XSLFShape shape : slide.getShapes()) {
if (shape instanceof XSLFTextShape) {
XSLFTextShape textShape = (XSLFTextShape) shape;
for (XSLFTextParagraph para : textShape) {
for (XSLFTextRun run : para) {
// 处理文本框中的动画
}
}
} else if (shape instanceof XSLFGroupShape) {
XSLFGroupShape groupShape = (XSLFGroupShape) shape;
for (XSLFShape subShape : groupShape.getShapes()) {
// 处理组合形状中的动画
}
} else {
// 处理其他形状中的动画
}
}
```
4. 获取每个XSLFShape对象中的动画,使用XSLFAnimationPainter类来渲染动画效果
```
XSLFAnimationPainter painter = shape.getAnimationPainter();
if (painter != null) {
painter.startAnimation();
// 处理动画效果
}
```
完整的Java代码示例如下:
```
import java.io.FileInputStream;
import java.io.IOException;
import org.apache.poi.xslf.usermodel.XMLSlideShow;
import org.apache.poi.xslf.usermodel.XSLFShape;
import org.apache.poi.xslf.usermodel.XSLFTextParagraph;
import org.apache.poi.xslf.usermodel.XSLFTextRun;
import org.apache.poi.xslf.usermodel.XSLFGroupShape;
import org.apache.poi.xslf.usermodel.XSLFTextShape;
import org.apache.poi.xslf.usermodel.XSLFSlide;
import org.apache.poi.xslf.usermodel.XSLFAnimationPainter;
public class PPTAnimationReader {
public static void main(String[] args) throws IOException {
FileInputStream fis = new FileInputStream("test.pptx");
XMLSlideShow ppt = new XMLSlideShow(fis);
for (XSLFSlide slide : ppt.getSlides()) {
for (XSLFShape shape : slide.getShapes()) {
if (shape instanceof XSLFTextShape) {
XSLFTextShape textShape = (XSLFTextShape) shape;
for (XSLFTextParagraph para : textShape) {
for (XSLFTextRun run : para) {
XSLFAnimationPainter painter = run.getAnimationPainter();
if (painter != null) {
painter.startAnimation();
// 处理动画效果
}
}
}
} else if (shape instanceof XSLFGroupShape) {
XSLFGroupShape groupShape = (XSLFGroupShape) shape;
for (XSLFShape subShape : groupShape.getShapes()) {
XSLFAnimationPainter painter = subShape.getAnimationPainter();
if (painter != null) {
painter.startAnimation();
// 处理动画效果
}
}
} else {
XSLFAnimationPainter painter = shape.getAnimationPainter();
if (painter != null) {
painter.startAnimation();
// 处理动画效果
}
}
}
}
ppt.close();
fis.close();
}
}
```
这里只是一个简单的示例,具体的动画处理需要根据实际情况来进行修改和完善。
阅读全文
相关推荐
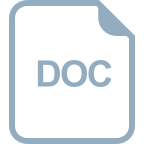
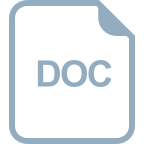
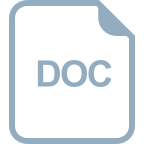


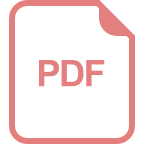
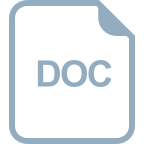
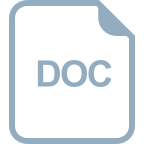
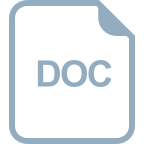
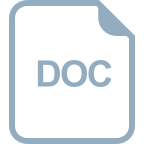
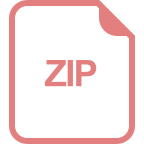