帮我写一个学生成绩查询统计系统的代码,要求有录入,排序,查找,统计功能
时间: 2024-03-20 09:42:11 浏览: 56
好的,以下是一个简单的学生成绩查询统计系统的代码,希望能帮到你:
```python
class Student:
def __init__(self, name, score):
self.name = name
self.score = score
class ScoreSystem:
def __init__(self):
self.students = []
def add_student(self, name, score):
student = Student(name, score)
self.students.append(student)
def sort_by_score(self):
self.students.sort(key=lambda x: x.score, reverse=True)
def search_student(self, name):
for student in self.students:
if student.name == name:
return student
return None
def get_average_score(self):
total_score = sum(student.score for student in self.students)
return total_score / len(self.students)
def get_max_score(self):
return max(student.score for student in self.students)
def get_min_score(self):
return min(student.score for student in self.students)
if __name__ == '__main__':
score_system = ScoreSystem()
score_system.add_student("张三", 80)
score_system.add_student("李四", 90)
score_system.add_student("王五", 85)
score_system.sort_by_score()
print("按成绩排序后:")
for student in score_system.students:
print(student.name, student.score)
search_name = "李四"
search_result = score_system.search_student(search_name)
if search_result:
print("查找到学生:", search_result.name, search_result.score)
else:
print("未找到学生:", search_name)
average_score = score_system.get_average_score()
print("平均分:", average_score)
max_score = score_system.get_max_score()
print("最高分:", max_score)
min_score = score_system.get_min_score()
print("最低分:", min_score)
```
代码中,我们定义了一个 `Student` 类来保存学生的姓名和成绩,以及一个 `ScoreSystem` 类来实现添加学生、按成绩排序、查找学生、统计平均分、最高分、最低分等功能。在 `main` 函数中,我们先添加了三个学生的信息,然后按成绩排序输出,接着查找李四的成绩,输出平均分、最高分、最低分等统计信息。
阅读全文
相关推荐
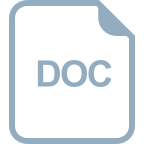
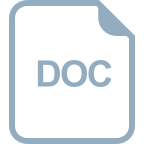
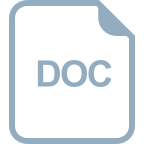















