写一段C语言代码示例为每个线程分配一个定时器操作
时间: 2024-02-25 15:52:54 浏览: 130
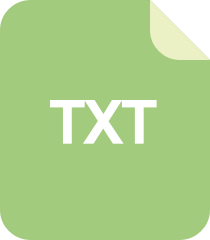
c语言有趣的100个例子代码

下面是一段使用timer_create为每个线程分配定时器的C语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <signal.h>
#include <time.h>
#define NUM_THREADS 4
void timer_handler(int signum) {
printf("Timer expired!\n");
}
void *thread_func(void *arg) {
timer_t timerid;
struct sigevent sev;
struct itimerspec its;
// Create the timer
sev.sigev_notify = SIGEV_SIGNAL;
sev.sigev_signo = SIGUSR1;
sev.sigev_value.sival_ptr = &timerid;
timer_create(CLOCK_REALTIME, &sev, &timerid);
// Set the timer
its.it_value.tv_sec = 1;
its.it_value.tv_nsec = 0;
its.it_interval.tv_sec = 1;
its.it_interval.tv_nsec = 0;
timer_settime(timerid, 0, &its, NULL);
// Wait for the timer to expire
sigset_t sigset;
sigemptyset(&sigset);
sigaddset(&sigset, SIGUSR1);
int sig;
sigwait(&sigset, &sig);
// Clean up the timer
timer_delete(timerid);
pthread_exit(NULL);
}
int main(int argc, char *argv[]) {
pthread_t threads[NUM_THREADS];
// Create the threads
for (int i = 0; i < NUM_THREADS; i++) {
pthread_create(&threads[i], NULL, thread_func, (void *)i);
}
// Wait for the threads to finish
for (int i = 0; i < NUM_THREADS; i++) {
pthread_join(threads[i], NULL);
}
return 0;
}
```
在上述代码中,主线程创建了NUM_THREADS个线程,并为每个线程分配了一个定时器。在每个线程中,首先使用timer_create创建了一个新的定时器,并将其附加到特定线程的ID上。然后使用timer_settime设置了定时器的初始值和间隔,并等待定时器到期。在等待过程中,该线程将阻塞,并等待SIGUSR1信号的到来。一旦定时器到期,SIGUSR1信号将被发送到该线程,并且信号处理函数timer_handler将被调用。在信号处理函数中,可以执行任何需要执行的操作,例如打印消息。最后,线程清理定时器并退出。
阅读全文
相关推荐
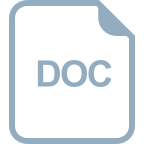
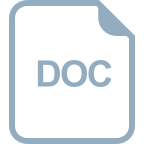
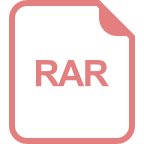
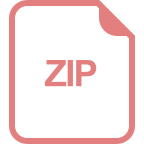
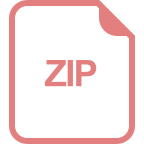
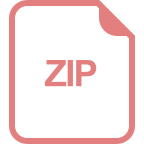
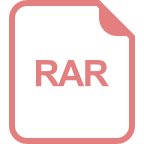
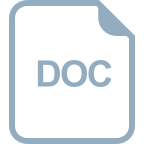
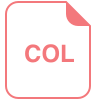
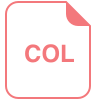
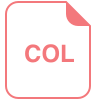
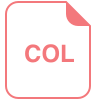
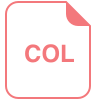
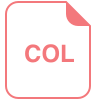
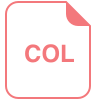
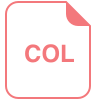
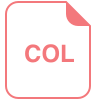
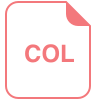