输入数字输出英文switch用c语言写数字范围在1~999之间
时间: 2024-10-15 12:20:14 浏览: 22
在C语言中,你可以使用`switch-case`结构配合`if-else`条件来处理数字并转换成对应的英文单词。由于数字1到999之间的范围较大,直接使用`switch`会变得复杂,因此我们可以将其拆分为三个部分:100以下、100到900以及1000。下面是一个简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
char* convert_to_english(int num) {
char* result;
if (num >= 1 && num <= 9) {
switch (num) {
case 1: result = "One"; break;
case 2: result = "Two"; break;
// ... 直至 case 9
}
} else if (num >= 10 && num <= 99) {
int tens = num / 10;
int units = num % 10;
char* tens_str = (tens == 1) ? "Ten" : (tens > 1 && tens < 10) ? "Tenths" : "Ty";
char* units_str = (units == 1) ? "One" : (units > 1 && units < 10) ? "Ones" : "Units";
result = malloc(strlen(tens_str) + strlen(units_str) + 3);
sprintf(result, "%s%s and %s", tens_str, (tens != 1 || units != 1) ? " " : "", units_str);
} else if (num == 100) {
result = "Hundred";
} else if (num >= 200 && num <= 900) {
int hundreds = num / 100;
int rest = num % 100;
char* hundreds_str = (hundreds == 1) ? "Hundred" : "Hundreds";
if (rest != 0) {
result = malloc(strlen(hundreds_str) + strlen(convert_to_english(rest)) + 4);
sprintf(result, "%s, %s", hundreds_str, convert_to_english(rest));
} else {
result = strdup(hundreds_str);
}
} else if (num == 999) {
result = "Nine Hundred Ninety-Nine";
} else {
result = "Number out of range!";
}
return result;
}
int main() {
int number;
printf("Enter a number between 1 and 999: ");
scanf("%d", &number);
char* english_num = convert_to_english(number);
printf("The English equivalent is: %s\n", english_num);
free(english_num); // Don't forget to free the allocated memory.
return 0;
}
```
这个程序首先检查输入的数字范围,然后使用不同的`switch`分支来处理各个区间内的数字。注意,对于100到999的部分,我们使用了递归的方式处理剩余的十位数。
阅读全文
相关推荐
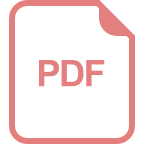
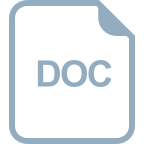
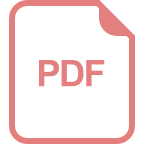















